To encrypt data programmatically in Android, you can use the Android cryptographic APIs. These APIs provide various encryption algorithms and methods to secure your data. By following the documentation and utilizing the appropriate classes and methods, you can implement encryption in your Android application.
In today’s digital age, data security is of utmost importance. With the proliferation of smartphones and the increasing amount of sensitive information stored on these devices, it is crucial to ensure that data is encrypted to protect it from unauthorized access. This is particularly true for Android devices, which are widely used worldwide.
Understanding Data Encryption
Data encryption refers to converting plain text into cipher text, making it unreadable to anyone without the proper decryption key. Encryption provides a layer of security that helps prevent unauthorized access and protects sensitive information from being compromised. In Android programming, data encryption can be implemented programmatically using various techniques.
What is Data Encryption?
Data encryption is converting plain text into cipher text to protect it from unauthorized access. Through encryption, data is transformed using an algorithm that requires a specific key to decrypt it back into its original form. This ensures that even if the encrypted data is intercepted, it cannot be read without the decryption key.
Data encryption has been used for centuries to protect sensitive information. In ancient times, military and government officials often used encryption to send secret messages. Today, encryption is widely used in various industries, such as finance, healthcare, and technology, to protect sensitive data from cyber threats.
There exist various kinds of encryption algorithms, which can be classified as symmetric and asymmetric encryption. Symmetric encryption involves the use of a single key for both encryption and decryption processes. On the other hand, asymmetric encryption employs a pair of keys, where the public key is used for encryption, and the private key is used for decryption.
Importance of Data Encryption in Android
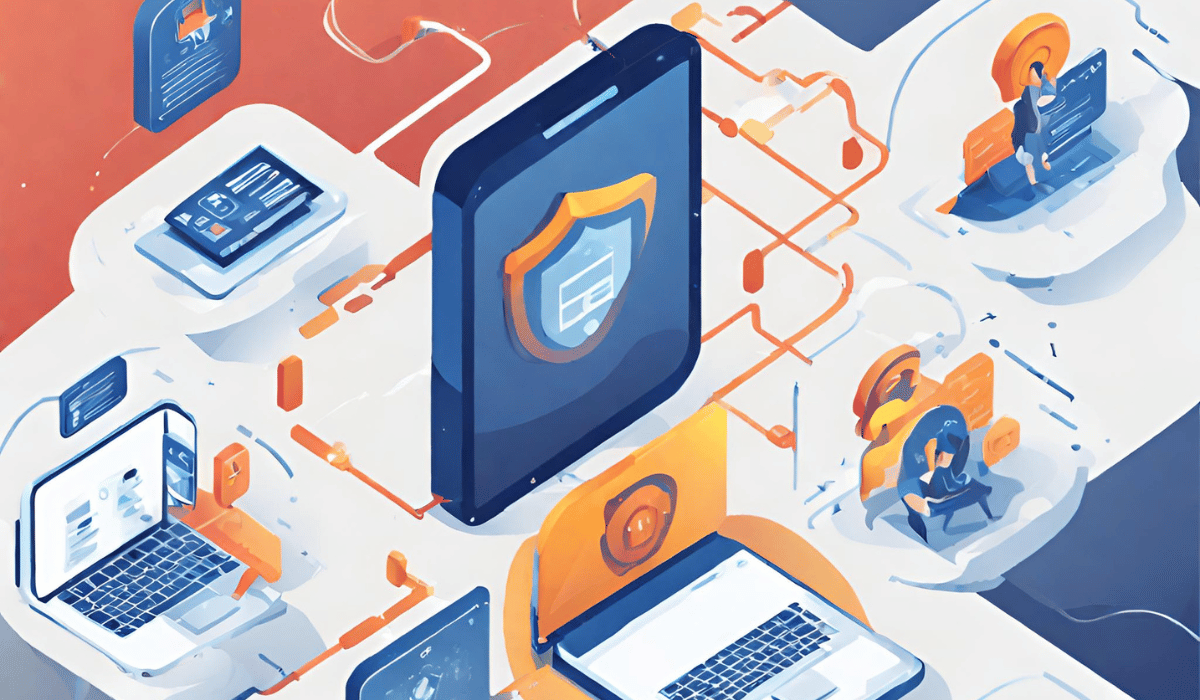
Android devices have become integral to our lives, storing personal and sensitive information. From emails and text messages to banking credentials and personal photos, our Android devices hold a treasure trove of data that needs to be protected. Data encryption is crucial in safeguarding this information from threats like data breaches, hacking attempts, or physical device theft.
Data encryption ensures that even if someone gains unauthorized access to the device, the encrypted data remains unreadable, mitigating the risk of sensitive information falling into the wrong hands.
Android provides various encryption techniques and APIs that developers can utilize to implement data encryption in their applications. One popular encryption technique is the Advanced Encryption Standard (AES), widely used for securing data on Android devices. AES is a symmetric encryption algorithm that provides high security and efficiency.
In addition to protecting data at rest, Android also supports encryption for data in transit. This means that data transmitted over the internet or other networks can be encrypted to prevent eavesdropping or interception by unauthorized parties.
Furthermore, Android devices offer features like secure key storage and hardware modules, which provide additional protection for encryption keys and sensitive data. These features ensure the encrypted data and keys are still secure even if an attacker gains physical access to the device.
Overall, data encryption in Android is essential for keeping the privacy and security of sensitive information stored on mobile devices. By implementing encryption techniques, developers can help protect user data and ensure it remains confidential, even in the face of potential threats.
Basics of Android Programming
Before diving into the intricacies of encrypting data programmatically in Android, it’s essential to have a solid foundation in Android programming. Let’s take a brief look at the key components of Android development.
Android programming is the procedure of creating applications for the Android operating system, which is used by billions of devices worldwide. It offers developers many possibilities to build innovative and interactive mobile applications.
Android Studio is a crucial asset in the field of Android programming. It is the designated integrated development environment (IDE) for creating Android applications. With its extensive utilities and functionalities, Android Studio streamlines developing, testing and troubleshooting Android apps.
Overview of Android Studio
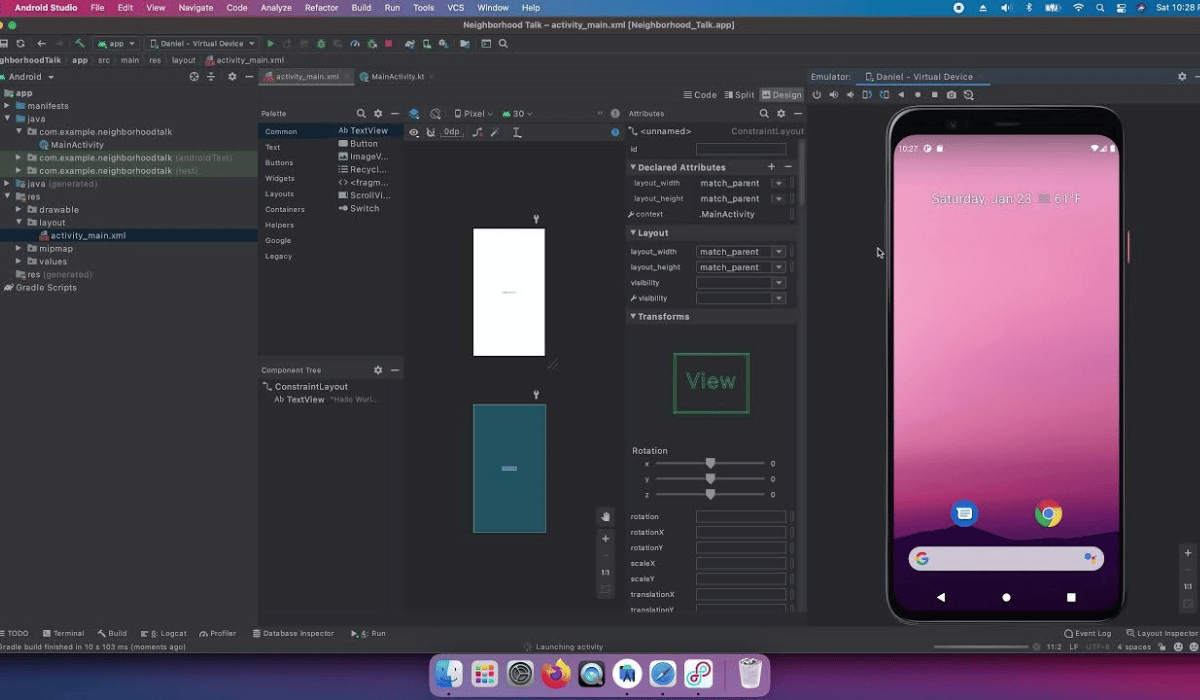
Android Studio offers a rich set of libraries, code templates, and an intuitive user interface, making it the preferred choice for most Android developers. It provides a visual editor for designing user interfaces, a powerful code editor with intelligent code completion, and a built-in emulator for testing apps on virtual devices.
With Android Studio, developers can easily create Android projects, manage dependencies, and build, run, and debug their applications. It also provides tools for performance profiling, memory analysis, and optimizing app performance.
Understanding Android SDK and APIs
The Android Software Development Kit (SDK) is a collection of software tools and libraries provided by Google to develop Android applications. Various APIs allow developers to access an Android device’s features and functionalities.
These APIs bridge your app and the underlying Android operating system, enabling you to leverage the device’s capabilities in your application. For example, you can use the camera API to capture photos or videos, the location API to get the device’s location, and the sensor API to access the device’s sensors, such as the accelerometer or gyroscope.
Furthermore, the Android SDK includes tools for building, testing, and debugging Android applications. It provides a command-line tool called ADB (Android Debug Bridge) for interacting with connected devices or emulators and tools for packaging and signing your app for distribution on the Google Play Store.
Understanding the Android SDK and APIs is crucial for Android programming, as they provide the necessary tools and resources to create powerful and feature-rich applications.
Setting Up Your Android Development Environment
Before encrypting data programmatically in Android, you must set up your development environment. This involves installing Android Studio and configuring the Android SDK.
Setting up your Android development environment ensures a smooth and efficient development process. Following the steps below, Android Studio will be installed and ready to use on your development machine and the necessary configurations to target specific Android versions and devices.
Installing Android Studio
To set up Android Studio, you can follow these easy steps:
- Start by visiting the official website of Android Studio and download the latest version. This guarantees that you have the most recent enhancements and features.
- Run the downloaded file and carefully follow the instructions displayed on your screen. Android Studio offers a user-friendly installation wizard that will guide you seamlessly.
- After the installation is finished, open Android Studio. You’ll be welcomed by a clean and intuitive interface that enhances your overall Android development experience.
Following these steps, Android Studio will be installed and ready to use on your development machine. Android Studio provides a comprehensive set of tools and features that simplify the development process, allowing you to focus on creating innovative and secure applications.
Configuring Android SDK
After installing Android Studio, you must configure the Android SDK to target the desired Android versions and devices. Here’s how:
- Open Android Studio and visit the “Preferences” or “Settings” menu. This menu provides access to various configuration options allowing you to customize your development environment according to your needs.
- Under the “Appearance & Behavior” section, select “System Settings” and then “Android SDK.” This will open the Android SDK Manager, where you can manage your installed SDK components.
- In the “SDK Platforms” tab, select the Android versions you want to target. It is essential to consider your target audience and their device specifications to ensure compatibility and optimal performance.
- Select the additional tools and components in the “SDK Tools” tab. Android Studio offers tools and libraries to enhance your development process, such as the Android Emulator, Android Debug Bridge (ADB), and Google Play services.
- Click “Apply” to save the changes and download the selected SDK components. Android Studio will automatically download and install the necessary SDK components, ensuring you have everything you need to develop your Android applications.
Once you have successfully configured the Android SDK, your development environment is ready to encrypt data programmatically in Android. The Android SDK provides a robust set of APIs and libraries that enable you to implement secure encryption algorithms and protect sensitive data within your applications.
Setting up your Android development environment is essential to becoming a proficient Android developer. Following these installation and configuration steps ensures you have the tools and resources to create high-quality and secure Android applications.
How To Encrypt Data Programmatically In Android
To encrypt data programmatically in Android, follow these steps:
-
Generate a Secret Key
The first step is to generate a secret key using the KeyGenerator class. Specify the algorithm, key size, and provider for key generation. The generated key will be used for encryption and decryption.
-
Initialize the Cipher
Create an instance of the Cipher class and initialize it with the appropriate transformation and secret key. The transformation specifies the encryption algorithm and mode of operation.
-
Encrypt the Data
Provide the plain text data to be encrypted and process it using the Cipher’s encryption mode. The encrypted data will be in the form of a byte array.
-
Store the Encrypted Data
Store the encrypted data securely, either in a file or a database. It is crucial to ensure that the storage location is adequately protected to prevent unauthorized access.
-
Decrypt the Data
Whenever you need to retrieve the data, follow a similar process but use the Cipher’s decryption mode instead. Provide the encrypted data; the Cipher will decrypt it using the secret key.
Storing and Retrieving Encrypted Data
When storing and retrieving encrypted data, it is essential to consider the following:
- Choose a secure storage location that provides adequate protection against unauthorized access.
- Use secure protocols and connections when transmitting encrypted data over networks.
- Implement proper authentication mechanisms to ensure that only authorized users can access the encrypted data.
Best Practices for Data Encryption
To ensure the effectiveness of data encryption in Android, consider the following best practices:
- Keep the encryption keys separate from the encrypted data.
- Use strong encryption algorithms and key sizes appropriate for the sensitivity of the data.
- Regularly update encryption libraries and dependencies to incorporate the latest security enhancements.
- Implement secure key management practices, such as key rotation and revocation.
- Test the encryption implementation thoroughly to identify and address any vulnerabilities.
Introduction to Android Security Architecture
Android takes security seriously and provides a robust security architecture to protect user data. Understanding the basics of Android security architecture is essential when developing secure Android applications.
Android Security Model
The Android security model is centered around sandboxing, which isolates each app’s data and resources from other apps. Each Android app runs in its sandbox, ensuring it cannot interfere with other apps or access their data without proper permission.
The Android security model also includes process isolation, permission-based access control, and cryptography to enhance privacy and protect sensitive data from unauthorized access.
Android’s Built-in Security Features
Android provides several built-in security features that developers can leverage when encrypting data programmatically. These features include:
- KeyStore: A secure container for storing cryptographic keys and certificates.
- Android Keystore System: A hardware-backed keystore implementation that provides additional security features.
- Secure Socket Layer (SSL)/Transport Layer Security (TLS): Protocols for establishing secure online communication channels.
- Android Permission System: A system that grants or denies access to resources based on user consent.
By utilizing these built-in security features, developers can reinforce data encryption in their Android apps, ensuring higher security and protection.
Data Encryption Techniques in Android
Data encryption in Android can be achieved using various techniques, depending on the specific requirements of your application. Let’s explore two commonly used Android encryption techniques: symmetric and asymmetric.
Symmetric Encryption
Symmetric encryption, also known as secret key encryption, uses a single key to encrypt and decrypt the data. This means the same key is used for encryption and decryption operations. Symmetric encryption algorithms, such as Advanced Encryption Standard (AES), provide fast and efficient encryption and decryption capabilities.
To encrypt data using symmetric encryption in Android, you generate a secret key and use it to encrypt the data. The same key is then used to decrypt the data when needed. The key should be securely stored to prevent unauthorized access.
Asymmetric Encryption
Asymmetric encryption, also known as public key encryption, uses a pair of keys: public and private keys. The public key is used for encryption, while the private key is used for decryption. This allows for secure communication between two parties without sharing a common key.
In Android, asymmetric encryption can be achieved using the Android Keystore System, which provides secure storage for cryptographic keys. The generated key pair can be used for encryption and decryption operations, ensuring the security and integrity of the data.
By combining symmetric and asymmetric encryption techniques, developers can create a secure and robust data encryption system in their Android applications.
Key Takeaways
- To encrypt data in Android programmatically, use the Android cryptographic APIs the Java Cryptography Architecture (JCA) provides.
- Choose an appropriate encryption algorithm, such as AES (Advanced Encryption Standard), and a secure mode of operation like CBC (Cipher Block Chaining).
- Generate a secure encryption key using a suitable key generation algorithm, such as AES KeyGenerator.
- Convert the data to be encrypted into a byte array.
- Initialize the encryption cipher with the encryption key and desired encryption parameters.
- Encrypt the data using the cipher’s encryption functionality.
- Store the encrypted data securely, such as in a file, SharedPreferences, or a secure database.
- Keep the encryption key secure and separate from the encrypted data.
FAQs
Can I use any encryption algorithm in Android?
Android supports various encryption algorithms through the JCA. Commonly used algorithms include AES, RSA, and DES. The choice of algorithm depends on factors such as security requirements, performance, and compatibility with other systems.
How can I securely store the encryption key?
To securely store the encryption key, consider using Android’s KeyStore system, which provides secure key storage and management. You can encrypt the key using a user-provided password or the Android Keystore system.
Can I encrypt data on external storage or SD cards?
Using Android’s built-in encryption mechanisms, you can encrypt data on external storage or SD cards. However, remember that the encryption may be tied to the specific device or SD card, and the encrypted data may not be accessible on other devices without decryption.
Conclusion
As technology continues to advance, the need for data encryption becomes increasingly crucial. Android developers must prioritize the security of their applications and implement data encryption programmatically. By understanding the basics of data encryption, setting up a suitable development environment, and leveraging the built-in security features of Android, developers can ensure the confidentiality and integrity of their users’ data. With the ever-growing threat landscape, staying updated with the latest encryption techniques and best practices to protect sensitive information on Android devices is imperative.