Importance of Data Security in Web Applications
Data security is of paramount importance in web applications for several reasons:
1. Protecting sensitive information: Web applications often handle sensitive user data such as personal information, financial details, and login credentials. Data breaches can lead to identity theft, financial loss, and other serious consequences for individuals. Implementing robust data security measures helps safeguard this information from unauthorized access.
2. Maintaining user trust: Users entrust web applications with their data, and it is crucial to ensure that their information is kept secure.
Understanding The Threat Landscape
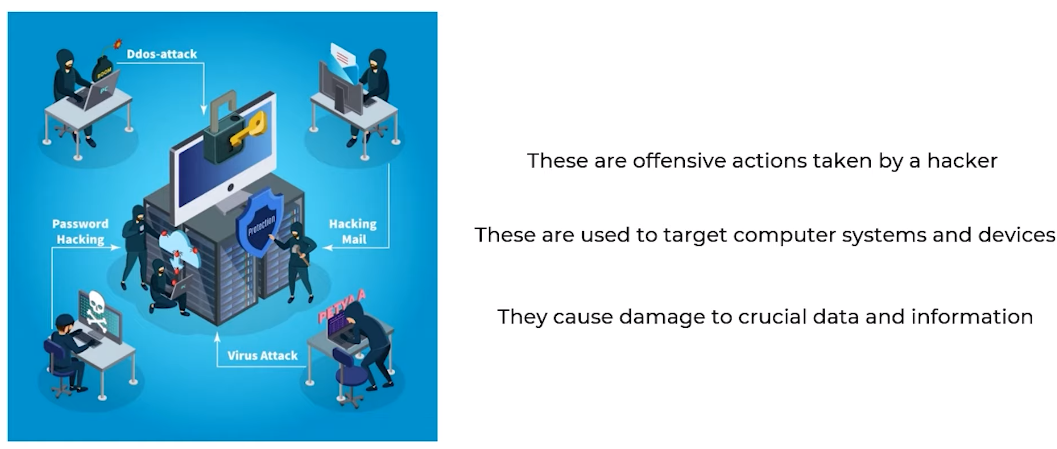
Understanding the threat landscape is essential for implementing effective data security measures. This involves staying informed about current and emerging threats, such as malware, phishing attacks, and social engineering tactics. By understanding the tactics used by malicious actors, web application developers can better protect against these threats.
Common Security Risks in Data Transmission
Common security risks in data transmission include:
1. Man-in-the-Middle Attacks: This occurs when a third party intercepts and potentially modifies the communication between two parties, without their knowledge. It can lead to unauthorized access to sensitive data.
2. Data Interception: This refers to the unauthorized access and capture of data during transmission. Attackers can use various techniques, such as packet sniffing, to intercept and steal sensitive information.
Potential Vulnerabilities in Data Transfer
Potential vulnerabilities in data transfer include:
1. Weak Encryption: If data is not properly encrypted during transmission, it can be easily intercepted and accessed by unauthorized individuals.
2. Insecure Protocols: The use of outdated or insecure protocols can make data transmission vulnerable to attacks. It is important to use secure protocols, such as HTTPS, to ensure the confidentiality and integrity of the data.
Angular’s Security Features
Angular, a popular JavaScript framework, provides several security features to protect against common vulnerabilities. Some of these features include:
1. Cross-Site Scripting (XSS) Protection: Angular automatically sanitizes user input to prevent XSS attacks. It ensures that any user input rendered in the browser is safe and does not execute malicious code.
2. Cross-Site Request Forgery (CSRF) Protection: Angular includes built-in mechanisms to prevent CSRF attacks. It generates and validates unique tokens for each request,
Angular’s Built-in Security Mechanisms
Angular includes several built-in security mechanisms to protect against common vulnerabilities. These mechanisms include:
1. Cross-Site Scripting (XSS) Protection: Angular automatically sanitizes user input to prevent XSS attacks. It ensures that any user input rendered in the browser is safe and does not execute malicious code.
2. Cross-Site Request Forgery (CSRF) Protection: Angular includes built-in mechanisms to prevent CSRF attacks.
How Angular Protects Against Common Security Threats?
Angular protects against common security threats through several mechanisms:
1. Input Sanitization: Angular automatically sanitizes user input to prevent Cross-Site Scripting (XSS) attacks. This ensures that any user input rendered in the browser is safe and does not execute malicious code.
2. Template Escaping: Angular escapes user-generated content in templates by default, preventing potential XSS attacks.
Role of Angular’s HTTP Module in Secure Data Transmission
The HTTP module in Angular plays a crucial role in ensuring secure data transmission. It provides features and mechanisms that help protect against common security threats, such as:
1. Secure Communication: The HTTP module supports HTTPS, allowing data to be transmitted over a secure connection. This helps prevent eavesdropping and tampering of sensitive information.
2. Encrypted Data: Angular’s HTTP module supports encryption protocols like SSL/TLS, which encrypts data during transmission, making it unreadable to unauthorized individuals.
Authentication and Authorization
Angular provides robust features for authentication and authorization, ensuring that only authorized users can access certain resources. Some of these features include:
1. User Authentication: Angular supports various authentication mechanisms, such as token-based authentication and OAuth, allowing users to securely log in and verify their identity.
2. Role-based Authorization: Angular allows developers to implement role-based authorization, where different users are granted different levels of access based on their roles or permissions.
Secure Data Transmission Techniques
Encryption
Some secure data transmission techniques include:
1. Encryption: Angular supports encryption techniques like SSL/TLS to ensure that data transmitted between the client and server is encrypted and cannot be easily intercepted or tampered with.
2. Secure Socket Layer (SSL)/Transport Layer Security (TLS): Angular supports SSL/TLS protocols to establish a secure connection between the client and server, encrypting the data in transit.
HTTPS Protocol
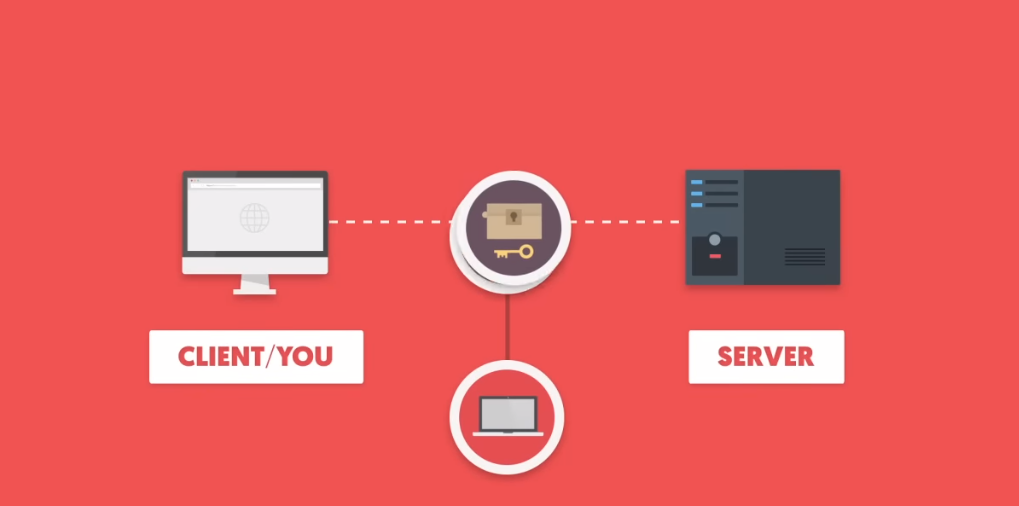
Some secure data transmission techniques include:
1. Encryption: Angular supports encryption techniques like SSL/TLS to ensure that data transmitted between the client and server is encrypted and cannot be easily intercepted or tampered with.
2. Secure Socket Layer (SSL)/Transport Layer Security (TLS): Angular supports SSL/TLS protocols to establish a secure connection between the client and server, encrypting the data in transit.
Cross-Site Scripting (XSS) Prevention
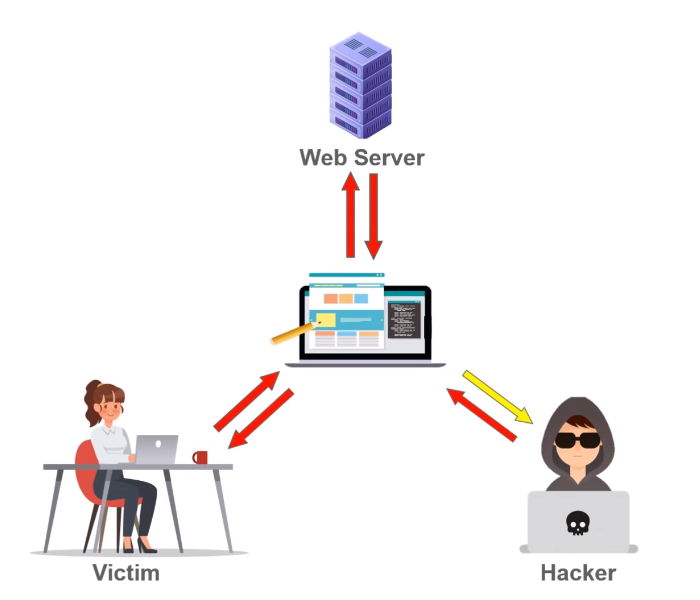
To prevent Cross-Site Scripting (XSS) attacks in Angular, you can follow these best practices:
1. Use Angular’s built-in security features: Angular provides built-in security mechanisms like template binding, sanitization, and content security policies (CSP) to prevent XSS attacks. Make sure to use these features properly to protect your application.
2. Sanitize user input: Always sanitize user input before rendering it in your application.
Cross-Site Request Forgery (CSRF) Protection
To prevent Cross-Site Request Forgery (CSRF) attacks in Angular, you can follow these best practices:
1. Use Angular’s built-in CSRF protection: Angular provides built-in mechanisms to protect against CSRF attacks. Make sure to enable and configure these features properly in your application.
2. Implement CSRF tokens: Use CSRF tokens in your application to verify the authenticity of requests. Include the CSRF token in all requests and validate it on the server side.
Content Security Policy (CSP)
To prevent Cross-Site Request Forgery (CSRF) attacks in Angular, you can also consider implementing Content Security Policy (CSP). CSP allows you to define a set of rules that restrict the types of content that can be loaded by your application, including external scripts and resources. By configuring CSP, you can mitigate the risk of malicious code injection and unauthorized resource loading.
Server-Side Input Validation
Server-side input validation is an important security measure to ensure that the data received from clients is valid and safe to process. It helps prevent various types of attacks, such as SQL injection and cross-site scripting (XSS).
Data Sanitization and Validation
Data sanitization and validation are crucial steps in ensuring the integrity and security of the data processed by an application. Sanitization involves removing or encoding potentially harmful characters or data from user input to prevent code injection or other malicious activities. Validation, on the other hand, verifies that the data meets certain criteria or constraints, such as format, length, or range, to ensure its validity and prevent unintended actions or errors.
Client-Side Data Validation
Client-side data validation is a technique used to validate user input directly on the client side, typically within a web browser, before sending the data to the server for further processing. This approach provides immediate feedback to the user and helps prevent unnecessary server requests. There are various ways to implement client-side data validation, such as using JavaScript frameworks or libraries, HTML5 form validation attributes, or custom JavaScript code.
Server-Side Data Validation
Server-side data validation is a technique used to validate user input on the server side after the data has been submitted from the client. This validation process ensures that the data meets the required criteria and is safe to be processed and stored. Server-side data validation is essential for maintaining data integrity and security, as it helps prevent malicious or incorrect data from being accepted and processed.
Error Handling and Logging
Error handling and logging are important aspects of server-side data validation. When an error occurs during the validation process, it is important to handle the error gracefully and provide meaningful feedback to the user. This can be done by displaying error messages or notifications, highlighting the fields that contain invalid data, and providing suggestions for correction. In addition to error handling, logging is also crucial for server-side data validation. Logging allows you to record any errors or exceptions that occur during the validation process.
Frequently Asked Questions (FAQs)
What is the significance of data security in web applications?
Data security is of utmost importance in web applications. It ensures the protection of sensitive information such as user credentials, personal data, and financial details. By implementing strong security measures, web applications can prevent unauthorized access, data breaches, and identity theft. This not only safeguards the privacy and trust of users but also helps businesses comply with legal and regulatory requirements. Additionally, data security helps maintain the integrity and reliability of the application, enhancing its overall reputation and user experience.
How does Angular ensure secure data transmission?
Angular ensures secure data transmission through several mechanisms:
1. HTTPS: Angular supports the use of HTTPS (Hypertext Transfer Protocol Secure) for secure communication between the client and server. HTTPS encrypts the data transmitted over the network, preventing unauthorized access and eavesdropping.
2. Cross-Site Scripting (XSS) Prevention: Angular provides built-in protection against Cross-Site Scripting attacks, which are a common vulnerability in web applications.
What are some common security risks in data transfer?
Some common security risks in data transfer include:
1. Man-in-the-Middle (MITM) Attacks: This type of attack occurs when an attacker intercepts the communication between two parties and can eavesdrop, modify, or inject malicious data into the transmission.
2. Data Breaches: Data breaches can occur when sensitive information is accessed or stolen by unauthorized individuals. This can happen due to weak encryption, improper handling of data, or vulnerabilities in the network.
How can I implement authentication and authorization in Angular?
To implement authentication and authorization in Angular, you can follow these steps:
1. Set up a backend server: You will need a backend server to handle authentication and authorization logic. This server will be responsible for validating user credentials, generating access tokens, and managing user roles and permissions.
2. Use a secure authentication method: Implement a secure authentication method, such as JSON Web Tokens (JWT), to authenticate users.
What is the role of encryption in secure data transmission?
Encryption plays a crucial role in secure data transmission by ensuring that the data being transmitted is protected from unauthorized access. It involves the process of converting plain text into cypher text using an encryption algorithm and a secret key. This cypher text can only be decrypted back to its original form using the corresponding decryption algorithm and key. When data is encrypted before transmission, it becomes difficult for attackers to intercept and understand the information being transmitted.
Why is the HTTPS protocol important for web applications?
The HTTPS protocol is important for web applications because it ensures secure communication between the user’s browser and the web server. It encrypts the data being transmitted, protecting it from unauthorized access and ensuring that it cannot be intercepted or tampered with by attackers. This helps to maintain the confidentiality and integrity of sensitive information, such as login credentials, personal data, and financial transactions.
How can I prevent XSS attacks in Angular?
To prevent XSS (Cross-Site Scripting) attacks in Angular, you can follow these best practices:1. Use Angular’s built-in security features: Angular provides several security mechanisms to protect against XSS attacks. For example, Angular’s template engine automatically escapes any potentially dangerous content, preventing it from being executed as code.
2. Sanitize user input: When accepting user input, make sure to properly sanitize and validate it before displaying it in your application.
Conclusion
In conclusion, error handling and logging are essential components of server-side data validation. By handling errors gracefully and providing meaningful feedback, users can be informed about any invalid data and guided towards making corrections. Logging, on the other hand, allows for the recording of errors and exceptions, providing valuable information for troubleshooting and debugging purposes. By implementing these practices, you can ensure a robust and reliable data validation process.