To encrypt data client-side in JavaScript, use a cryptographic library like Web Crypto API or CryptoJS to implement encryption algorithms such as AES or RSA. Generate a secure key and encrypt the data locally on the client-side before sending it to the server. Remember not to store the encryption key or sensitive data in plaintext.
In today’s digital age, data privacy has become a pressing concern. As technology advances, so do the techniques hackers and malicious entities use to access sensitive information. This has led to an increased demand for secure methods of data encryption. One such method is client-side encryption, performed on the client’s device before the data is transmitted to a server.
Understanding Encryption in JavaScript
Encryption is the process of encoding data so that only authorized parties can access it. In JavaScript, encryption can be achieved using various algorithms and libraries. Before diving into the implementation details, it is important to grasp the basics of encryption.
Encryption relies on algorithms that transform plain text into cipher text, which is the encrypted form of the data. These algorithms use a key, a parameter that determines the transformation. To successfully decrypt the data, the recipient needs to have the same key used for encryption. This ensures that only authorized parties can access the decrypted information.
Several commonly used encryption algorithms in JavaScript, such as AES (Advanced Encryption Standard), RSA (Rivest-Shamir-Adleman), and Blowfish. Each algorithm has its characteristics and level of security. AES, for example, is widely used and considered secure for most applications. Conversely, RSA is commonly used for asymmetric encryption, where different keys are used for encryption and decryption.
Basics of Encryption
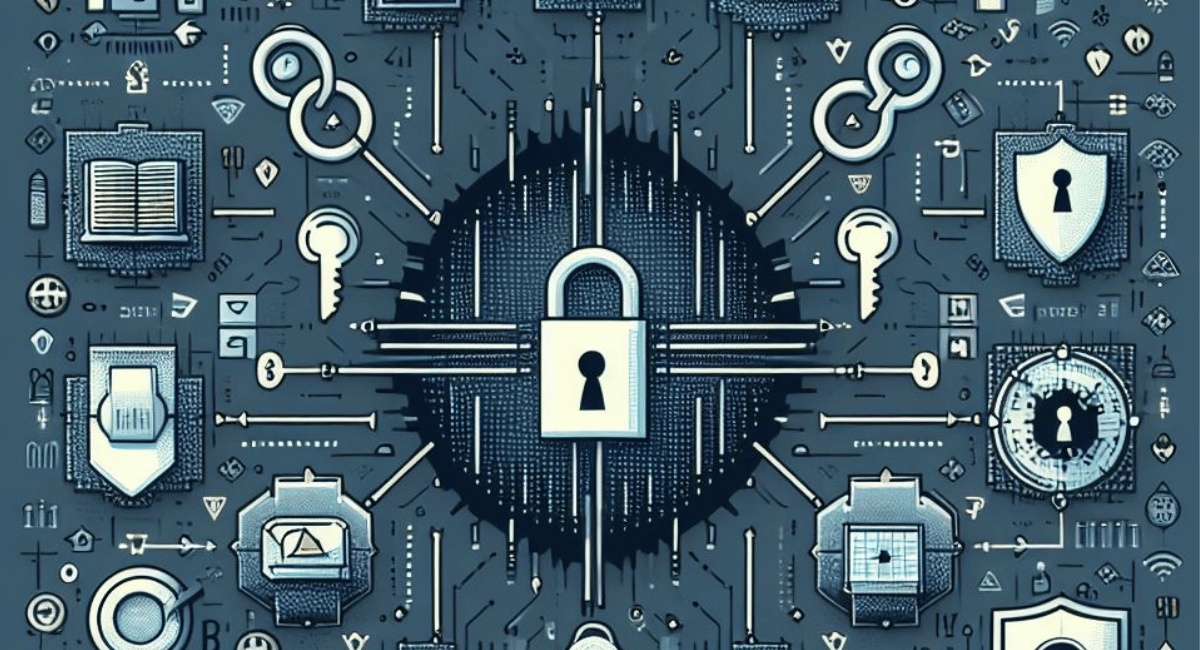
Encryption is a fundamental concept in information security. It protects sensitive data, such as passwords, credit card information, and personal messages, from unauthorized access. Encrypting data, even if it is intercepted or accessed by an unauthorized party, will appear gibberish without the proper decryption key.
Encryption algorithms can be broadly categorized into two types: symmetric and asymmetric. Symmetric encryption algorithms use the same key for encryption and decryption, while asymmetric encryption algorithms use different keys for encryption and decryption.
One common symmetric encryption algorithm is the Data Encryption Standard (DES), which has been widely used but is now considered relatively weak due to advances in computing power. AES, a more secure and widely adopted symmetric encryption algorithm, has replaced DES in many applications.
Asymmetric encryption algorithms, such as RSA, use a pair of keys: a public key for encryption and a private key for decryption. The public key can be freely shared with anyone, while the private key must be kept secret. When someone wants to send an encrypted message to the public key owner, they use the public key to encrypt the message. The owner of the private key can then decrypt the message using the private key.
Importance of Client-Side Encryption
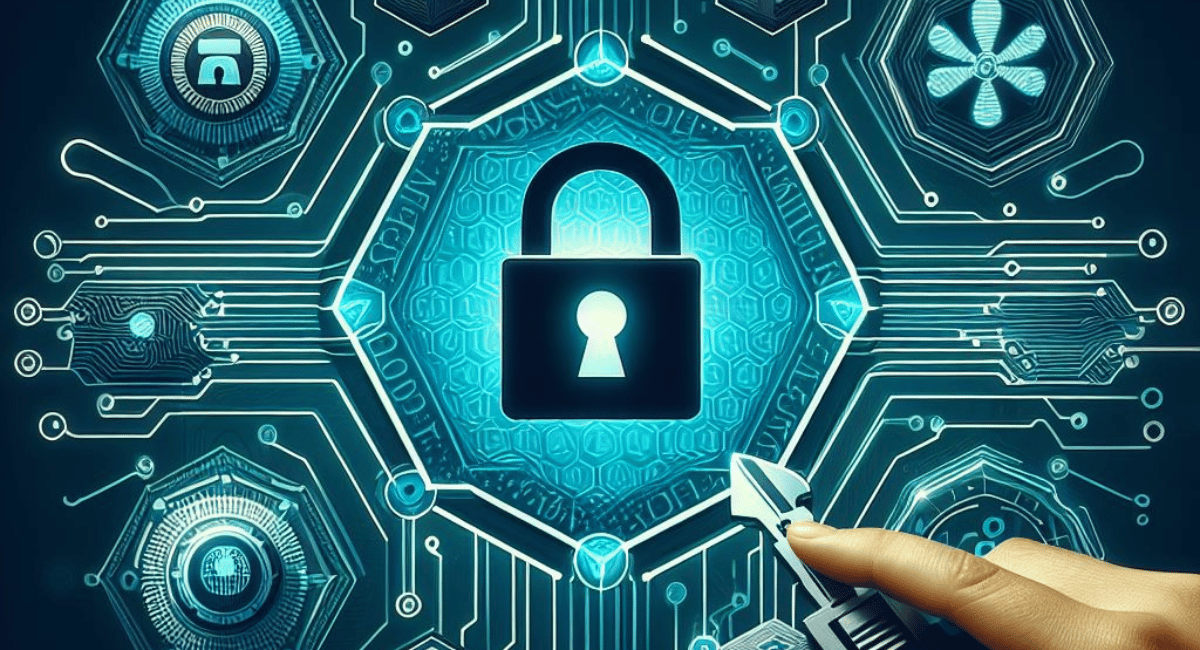
Client-side encryption offers an additional layer of security by encrypting the data before it even leaves the client’s device. Traditional server-side encryption can be vulnerable to attacks where the encrypted data is intercepted during transmission. Client-side encryption mitigates this risk by ensuring the data is encrypted locally before leaving the client’s device.
With client-side encryption, the data is encrypted using the client’s encryption keys, which are never transmitted to the server. This means that the encrypted data remains secure even if the server is compromised or hacked. Only the client with the decryption keys can access the decrypted information.
Client-side encryption is particularly important in scenarios where sensitive data needs to be stored in the cloud or transmitted over the internet. Encrypting the data on the client’s device significantly reduces the risk of unauthorized access or data breaches.
It is worth noting that while client-side encryption enhances security, it also introduces some challenges. For example, managing encryption keys securely becomes crucial. The encryption and decryption processes can also consume more computational resources on the client’s device, potentially impacting performance.
In conclusion, understanding encryption in JavaScript is essential for building secure web applications. Developers can protect sensitive data by implementing encryption algorithms and client-side encryption techniques and ensure that only authorized parties can access it.
Setting Up Your JavaScript Environment
Before implementing encryption in JavaScript, we need to set up the necessary tools and libraries.
Setting up your JavaScript environment for encryption involves installing the required tools and libraries. One of the most popular libraries for encryption in JavaScript is Crypto-JS. Crypto-JS provides a wide range of cryptographic functions that can be used for encryption, decryption, and other related operations.
To install Crypto-JS, you can use npm, the Node.js package manager. npm is a command-line tool that allows you to install, manage, and share JavaScript packages. It comes bundled with Node.js, so you need to have Node.js installed on your system before you can use npm.
If you don’t have Node.js installed, you can download it from the official Node.js website and follow the installation instructions for your operating system.
Once you have Node.js installed, you can open your command-line interface and run the following command to install Crypto-JS:
npm install crypto-js
This command will download the Crypto-JS package from the npm registry and install it in your project’s node_modules directory. The node_modules directory is where npm stores all the packages you install for your project.
After the installation is complete, you can import Crypto-JS into your JavaScript file using the required function:
const CryptoJS = require(‘crypto-js’);
This line of code imports the Crypto-JS module and assigns it to the variable CryptoJS. You can now use the CryptoJS object to access the cryptographic functions provided by Crypto-JS.
Setting up your JavaScript environment for encryption is an important step in ensuring the security of your data. Using a reliable and widely-used library like Crypto-JS, you can leverage the power of cryptography in your JavaScript applications.
Necessary Tools and Libraries
One of the most popular libraries for encryption in JavaScript is Crypto-JS. Crypto-JS provides a wide range of cryptographic functions that can be used for encryption, decryption, and other related operations. To install Crypto-JS, you can use npm, the Node.js package manager.
Implementing Basic Encryption
Now that our JavaScript environment is set up, we can implement basic encryption using Crypto-JS.
Encryption is a fundamental technique to secure data by converting it into an unreadable format. This ensures that even if the encrypted data is intercepted, it cannot be understood without the corresponding decryption key.
Writing the Encryption Function
The first step in encrypting data is to write an encryption function. This function takes the plain text data and the encryption key as input and returns the encrypted cipher text. The encryption algorithm used by Crypto-JS is AES (Advanced Encryption Standard), which is a widely accepted encryption standard.
When encrypting data using AES, the plain text is divided into blocks, and each block is encrypted separately. This adds an extra layer of security to the encryption process.
Understanding Key Generation
A crucial aspect of encryption is key generation. The encryption key needs to be unpredictable and unique for each encryption operation. Crypto-JS provides built-in functions to generate random and secure encryption keys.
Key generation involves using algorithms to create a sequence of random bits that form the encryption key. These algorithms ensure the generated key is highly secure and cannot be easily guessed or reverse-engineered.
Generating a new key for each encryption operation is important to prevent unauthorized access to the encrypted data. Reusing the same key for multiple encryption operations can compromise the security of the encrypted data.
Advanced Encryption Techniques
While basic encryption provides a good level of security, there are advanced techniques that can further enhance data protection in JavaScript.
When it comes to securing sensitive data, encryption plays a crucial role. It ensures that information remains confidential and inaccessible to unauthorized individuals. In JavaScript, several advanced encryption techniques go beyond the basic methods.
Using Public and Private Keys
Public-key encryption, also known as asymmetric encryption, involves using two keys: a public key for encryption and a private one for decryption. This technique provides an added layer of security as the public key can be freely shared while the private key remains confidential.
When public and private keys are used, the encryption process begins with the recipient generating a key pair. The public key is then shared with the sender, who uses it to encrypt the data before transmitting it. Once the encrypted data is received, the recipient can decrypt it using their private key, which is securely stored and kept secret.
This encryption method is widely used in various applications, such as secure email communication, digital signatures, and secure online transactions. It ensures that sensitive information remains secure, even if intercepted by malicious actors.
Implementing AES Encryption
AES encryption is a symmetric encryption algorithm that uses the same key for encryption and decryption. It is known for its efficiency and security. By implementing AES encryption in JavaScript, we can ensure that our data remains secure despite advanced attacks.
The Advanced Encryption Standard (AES) has become the most widely used symmetric encryption algorithm. It provides a high level of security and is resistant to various cryptographic attacks. AES encryption operates on fixed block sizes, typically 128 bits, and supports key sizes of 128, 192, and 256 bits.
When implementing AES encryption in JavaScript, developers can use various libraries and frameworks that provide robust cryptographic functions. These libraries ensure that the encryption process is performed efficiently and securely, minimizing the risk of vulnerabilities.
Using AES encryption, JavaScript applications can protect sensitive data, such as passwords, credit card information, and personal details. This added layer of security instills confidence in users, knowing that their information is safeguarded against unauthorized access.
Furthermore, AES encryption can be combined with other security measures, such as secure key management and communication protocols, to create a comprehensive security framework. This ensures that data is protected during transmission and at rest.
In conclusion, advanced encryption techniques, such as public and private key encryption and AES encryption, provide enhanced data protection in JavaScript. By implementing these techniques, developers can ensure the security and confidentiality of sensitive information, mitigating the risks associated with unauthorized access and data breaches.
Decrypting Data in JavaScript
Decrypting encrypted data in JavaScript is as important as encrypting it. Let’s explore how we can write a decryption function.
Writing the Decryption Function
The decryption function takes the cipher text and the decryption key as input and returns the decrypted plain text. It utilizes the same encryption algorithm as the encryption function but with the decryption key instead.
Handling Errors and Exceptions
During the decryption process, errors and exceptions may occur. It is important to handle them appropriately to ensure the smooth flow of the decryption process. We can provide a more robust encryption and decryption system by implementing proper error handling.
Key Takeaways
- Client-side encryption in JavaScript enhances data security by encrypting data before transmission.
- Libraries like “crypto-js” can be used to implement encryption in JavaScript.
- Encryption keys need to be stored securely and should never be exposed.
- Server-side decryption is a complementary process, requiring the same keys to decrypt data for use or storage.
- Properly implemented, client-side encryption can significantly reduce the risk of data being intercepted or stolen during transmission.
FAQs
What is client-side encryption in JavaScript?
Client-side encryption refers to data being encrypted in the client’s browser before being sent to a server. It’s usually done using JavaScript libraries that support encryption.
How can I encrypt data in JavaScript?
Data encryption can be achieved in JavaScript using various libraries, for example, the “crypto-js” library, which supports AES, MD5, SHA-1, etc.
Is it safe to encrypt data on the client side?
Client-side encryption is typically secure as the data is encrypted before leaving the client’s system. However, encryption keys should never be exposed or stored insecurely.
How does client-side encryption work with server-side decryption?
After the data is encrypted on the client side, it’s sent to the server. The server uses the correct decryption key to decrypt the data for use or storage.
What is the best practice for storing encryption keys?
Encryption keys should be stored securely and not exposed. Ideally, they should be stored in a secure server environment or using a key management service.
Conclusion
In conclusion, encrypting data on the client side using JavaScript effectively enhances data security. By understanding the basics of encryption, setting up the necessary tools and libraries, and implementing both basic and advanced encryption techniques, we can ensure that our data remains secure throughout its lifecycle. Decrypting the data in JavaScript is equally important, and by following proper decryption practices, we can maintain data integrity and protect sensitive information from unauthorized access. As technology continues to evolve, client-side encryption will play a crucial role in safeguarding our data in the digital world.