Java offers robust tools and libraries for data encryption. You can leverage Java’s Cipher class along with encryption algorithms like AES, DES, and RSA to implement secure encryption processes. Additionally, Java provides key management classes to handle encryption keys securely, ensuring a comprehensive approach to data security.
Data encryption is a crucial aspect of secure data handling in any software application. In this article, we will explore how to implement data encryption in Java, a widely used programming language. By understanding the basics of data encryption, setting up the necessary tools for encryption, learning about different encryption techniques available in Java, and implementing encryption in your projects, you will be equipped to protect sensitive data effectively.
Understanding Data Encryption
Data encryption is the process of converting plain text data into an unreadable format, commonly referred to as ciphertext. This transformation ensures that even if an unauthorized person gains access to the data, they will not be able to understand it without the corresponding decryption key. Encryption plays a critical role in safeguarding data privacy and confidentiality.
The Importance of Data Encryption
In today’s digital age, where data breaches and cyber-attacks are rampant, encrypting sensitive information is of utmost importance. Whether it’s personal data, financial transactions, or confidential business information, encryption ensures that data remains secure and protected from unauthorized access.
Data encryption provides a layer of defense against malicious actors who may try to intercept or manipulate data during transmission. By encrypting data, organizations can mitigate the risk of data breaches and unauthorized access, safeguarding their reputation and maintaining the trust of their customers and stakeholders.
Furthermore, data encryption is crucial for compliance with various data protection regulations, such as the General Data Protection Regulation (GDPR) and the Health Insurance Portability and Accountability Act (HIPAA). These regulations require organizations to implement appropriate security measures, including encryption, to protect sensitive data.
Basics of Data Encryption in Java
Java provides rich libraries and APIs that simplify the implementation of data encryption. To encrypt data in Java, developers can leverage encryption algorithms and cryptographic techniques provided by the Java Cryptography Architecture (JCA).
Encryption in Java can be achieved through both symmetric and asymmetric encryption techniques. Symmetric encryption uses a single key for both encryption and decryption, while asymmetric encryption uses a pair of mathematically related keys – a public key for encryption and a private key for decryption.
When implementing data encryption in Java, developers have the flexibility to choose from a wide range of encryption algorithms, such as Advanced Encryption Standard (AES), Data Encryption Standard (DES), and Rivest Cipher (RC4). These algorithms offer different levels of security and performance, allowing developers to select the most appropriate one based on their specific requirements.
In addition to encryption algorithms, Java also provides support for key management, secure key exchange protocols, and digital signatures, further enhancing the security of encrypted data. Developers can generate, store, and manage encryption keys securely, ensuring the integrity and confidentiality of sensitive information.
Java’s encryption capabilities extend beyond just text data. It can also encrypt files, databases, and network communications, providing a comprehensive solution for protecting data at rest and in transit.
Overall, Java’s robust encryption features empower developers to implement strong data protection measures, ensuring the security and privacy of sensitive information in various applications and systems.
Setting Up Your Java Environment for Encryption
Before diving into encryption in Java, you need to set up the required tools and libraries to ensure a smooth implementation process.
Setting up your Java environment for encryption involves installing and configuring the necessary tools and libraries. This ensures that you have everything you need to successfully implement encryption in your Java applications.
Required Tools and Libraries
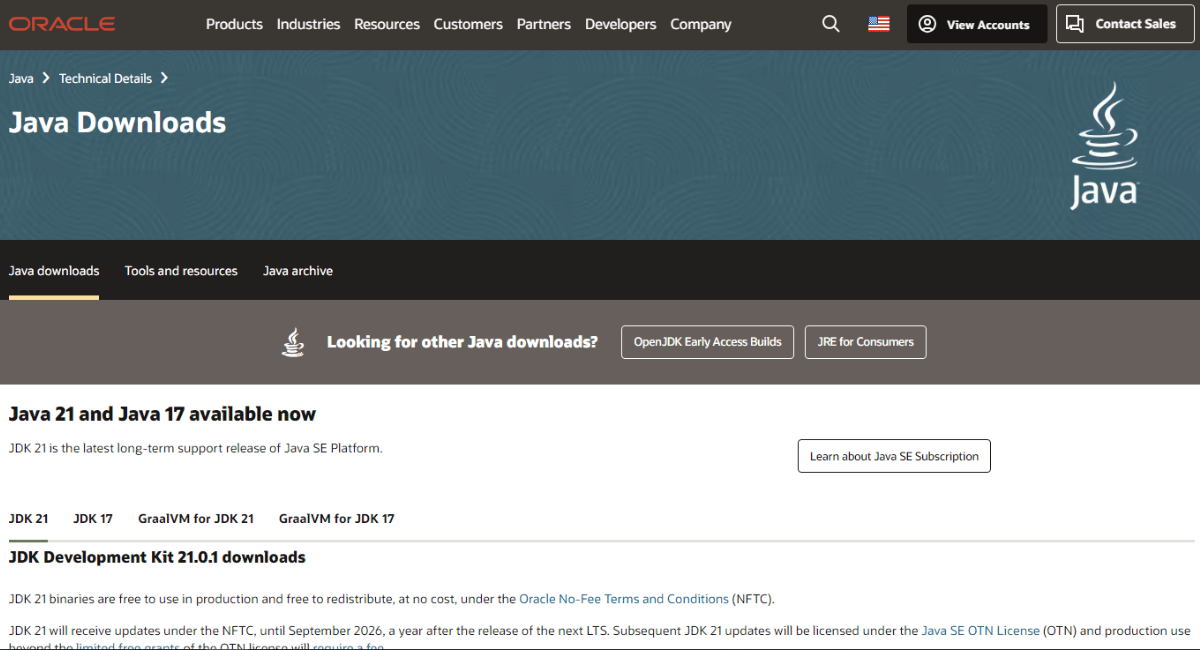
To get started with encryption in Java, you’ll need:
- Java Development Kit (JDK)
- Java Cryptography Extension (JCE)
The JDK provides the necessary development tools and runtime environment for Java applications. It includes the Java compiler, runtime libraries, and other tools that are essential for developing and running Java programs.
The JCE, on the other hand, is a Java extension that supports various encryption algorithms, including AES (Advanced Encryption Standard) and DES (Data Encryption Standard). It provides the cryptographic functions and algorithms necessary for implementing encryption and decryption in Java applications.
Installing and Configuring Java Cryptography Extension (JCE)
The JCE comes bundled with Java SE, but depending on the version of Java you are using, you may need to install and configure it separately. This ensures that you have the latest version of the JCE and any additional security updates.
To install the JCE, you can download the JCE files from the official Oracle website. The download page provides instructions on how to install and configure the JCE for your specific version of Java.
Once you have downloaded the JCE files, you can follow the installation instructions provided to install and configure the JCE. These instructions may vary depending on your operating system and Java version.
After successfully installing and configuring the JCE, you will have access to the additional encryption algorithms and functions provided by the JCE. This allows you to implement more secure and robust encryption in your Java applications.
Setting up your Java environment for encryption is an important step in ensuring the security of your applications. By installing and configuring the necessary tools and libraries, you can leverage the power of encryption to protect sensitive data and ensure the privacy and integrity of your information.
Java Encryption Techniques
Java provides developers with versatile encryption techniques to fit different security requirements. Encryption is a crucial aspect of modern-day computing, ensuring the confidentiality and integrity of sensitive data.
When it comes to encryption in Java, there are two primary categories: symmetric encryption and asymmetric encryption. Each category has its own set of algorithms and use cases.
Symmetric Encryption in Java
Symmetric encryption is the simplest form of encryption and involves using the same key for both encryption and decryption. Java supports various symmetric encryption algorithms, such as Advanced Encryption Standard (AES), Data Encryption Standard (DES), and Triple DES (3DES).
The process of symmetric encryption in Java involves several steps. First, you need to generate a secret key, which is a random sequence of bits. This key will be used to encrypt and decrypt the data. Next, you need to provide the necessary padding and mode of operation. Padding ensures that the data is properly aligned and can be encrypted in blocks, while the mode of operation determines how the encryption algorithm processes the data. Finally, you apply the encryption algorithm to your data, transforming it into an unreadable format.
One advantage of symmetric encryption is its speed and efficiency. Since the same key is used for both encryption and decryption, the process is relatively fast. However, the key needs to be securely shared between the sender and the recipient.
Asymmetric Encryption in Java
Asymmetric encryption, also known as public-key encryption, uses a pair of mathematically related keys – a public key for encryption and a private key for decryption. Java supports asymmetric encryption using algorithms like RSA and Elliptic Curve Cryptography (ECC).
The process of implementing asymmetric encryption in Java involves several steps. First, you need to generate a key pair consisting of a public key and a private key. The public key is shared with others, while the private key is kept secret. When someone wants to send you an encrypted message, they use your public key to encrypt it. Only you, with your private key, can decrypt and read the message.
One advantage of asymmetric encryption is its ability to establish secure communication channels without the need for pre-shared keys. It enables secure key exchange and digital signatures, ensuring the authenticity and integrity of data.
However, asymmetric encryption is computationally expensive compared to symmetric encryption. The encryption and decryption processes involve complex mathematical operations, making it slower than symmetric encryption. As a result, it is often used for securing sensitive information like passwords, digital certificates, and secure communication protocols.
Overall, Java provides developers with a comprehensive set of encryption techniques to meet various security requirements. Whether you need to encrypt sensitive data using symmetric encryption or establish secure communication channels using asymmetric encryption, Java has the necessary tools and algorithms to ensure the confidentiality and integrity of your information.
Implementing Data Encryption in Java
Now that you have the necessary knowledge about data encryption and the tools required in the Java environment, it’s time to implement encryption in your projects.
Writing the Encryption Code
First, you need to write the encryption code, which involves selecting an encryption algorithm, generating a secret key or key pair, and configuring the encryption parameters such as padding and mode of operation.
Using the Java encryption libraries and APIs, you can easily create an instance of the chosen algorithm, generate the necessary encryption keys, and configure the cipher settings.
Encrypting and Decrypting Data
With the encryption code in place, you can now encrypt and decrypt your data. Using the generated encryption key(s) and the cipher instance, you can encrypt sensitive information, such as passwords, credit card details, or personal data.
Decryption follows a similar process, where the encrypted data and the corresponding decryption key(s) are used to retrieve the original plain text.
Common Encryption Algorithms in Java
Java provides several encryption algorithms to choose from, depending on your specific security requirements. Let’s explore some of the commonly used encryption algorithms in Java.
Advanced Encryption Standard (AES)
AES is a widely accepted symmetric encryption algorithm that offers strong security and performance. It supports key sizes of 128, 192, and 256 bits, making it suitable for a broad range of applications.
In Java, the AES algorithm is available in the Java Cryptography Architecture (JCA), allowing developers to incorporate AES encryption seamlessly into their code.
Data Encryption Standard (DES)
DES is a symmetric encryption algorithm widely used but now considered less secure due to its small key size. Nevertheless, it can still be used for certain low-security applications. Java provides support for both DES and Triple DES (3DES) encryption.
When using DES in Java, it is important to note that higher key sizes and more secure algorithms like AES are recommended for better security.