To encrypt JSON data in Java, you can use libraries like Javax.Crypto or Bouncy Castle. These libraries provide methods to encrypt and decrypt data using various encryption algorithms such as AES or RSA. Simply convert your JSON data into a string, apply the encryption algorithm with a secret key or public key, and store the encrypted data.
I will explore how to encrypt JSON data in Java. Encryption is a crucial aspect of securing sensitive information, and by understanding the basics of encryption and learning how to apply it to JSON data in Java, you can enhance the security of your applications.
Understanding Encryption and Its Importance
Encryption is a critical aspect of data security in the digital age. It involves the process of converting data into a format that is unreadable or meaningless to unauthorized individuals. Encryption transforms data into ciphertext by utilizing cryptographic algorithms and keys, making it difficult for unauthorized parties to decipher.
But what exactly is encryption, and why is it necessary?
What is Encryption?
Encryption is a sophisticated technique that ensures data confidentiality, integrity, and authenticity. It protects sensitive information from being accessed by malicious entities. Through encryption, data is transformed into an unreadable form, known as ciphertext, which can only be decrypted using the corresponding decryption key.
Encryption algorithms are designed to be highly secure, making it extremely challenging for unauthorized individuals to break the encryption and gain access to the original data. This process protects sensitive information, such as financial transactions, personal data, and confidential business records.
Why is Encryption Necessary?
Data breaches and security threats are a constant concern in today’s digital landscape. Encryption is necessary to safeguard data privacy and protect sensitive information from unauthorized access. It is a powerful defense mechanism against cybercriminals, hackers, and malicious actors.
By encrypting data, even if it is intercepted during transmission or stored on a compromised device, it remains inaccessible and unintelligible to unauthorized individuals. This ensures that sensitive information remains secure and confidential, mitigating the risk of data breaches and unauthorized access.
Moreover, encryption plays a vital role in ensuring the integrity and authenticity of data. With encryption, any unauthorized modifications or tampering attempts can be easily detected. This helps maintain the trustworthiness of the data and ensures that it has not been altered or manipulated.
Encryption is crucial in industries that handle highly sensitive data, such as healthcare, finance, and government. It helps organizations comply with data protection regulations and industry standards, safeguarding sensitive information.
Furthermore, encryption is not limited to protecting data at rest or in transit. It also extends to securing data stored on devices like laptops, smartphones, and external hard drives. In the event of theft or loss, encrypted data remains protected, preventing unauthorized access to sensitive information.
Overall, encryption is an essential tool in the fight against cyber threats and data breaches. By implementing strong encryption measures, individuals and organizations can enhance data security, maintain privacy, and preserve the integrity of their information.
Basics of JSON and Java
Introduction to JSON
JSON (JavaScript Object Notation) is a lightweight data-interchange format used by modern applications to transmit and store data. It is human-readable and easy to parse, making it a popular choice for data exchange between systems. JSON data consists of key-value pairs organized into objects, arrays, and nested structures.
When working with JSON, it’s important to understand its simplicity and flexibility. Unlike other data formats, such as XML, JSON has a more concise syntax, making it easier to read and write. It is based on a subset of JavaScript, which means that it can be easily understood by developers familiar with the language.
One of the key advantages of JSON is its compatibility with a wide range of programming languages. It is natively supported by most modern programming languages, including Java, making integrating JSON data into your applications easy.
JSON supports various data types, including strings, numbers, booleans, arrays, objects, and null values. This versatility allows developers to represent complex data structures straightforwardly and intuitively.
Understanding Java
Java is a widely-used programming language known for its platform independence and versatility. It provides a rich set of libraries and APIs, simplifying the development of secure and robust applications. Java is suitable for handling JSON data due to its extensive support for JSON processing, including parsing, manipulation, and serialization.
When working with JSON in Java, developers can leverage libraries such as Jackson, Gson, or JSON.simple to parse and generate JSON data. These libraries provide easy-to-use APIs that abstract the complexities of JSON processing, allowing developers to focus on their application logic.
Java’s object-oriented nature makes it a natural fit for working with JSON data. Developers can easily map JSON objects to Java classes using frameworks like Jackson or Gson, allowing seamless integration between JSON and Java objects. This makes it straightforward to manipulate and transform JSON data within a Java application.
Furthermore, Java provides robust error-handling mechanisms essential for external data sources like JSON. Exception handling in Java allows developers to gracefully handle errors and ensure the stability and reliability of their applications.
Another advantage of using Java for JSON processing is its performance. Java’s Just-In-Time (JIT) compilation and optimized runtime environment make it a fast and efficient choice for handling large volumes of JSON data. This is particularly important in scenarios requiring real-time processing or high throughput.
Tools Required for Encrypting JSON Data in Java
Java Development Kit (JDK)
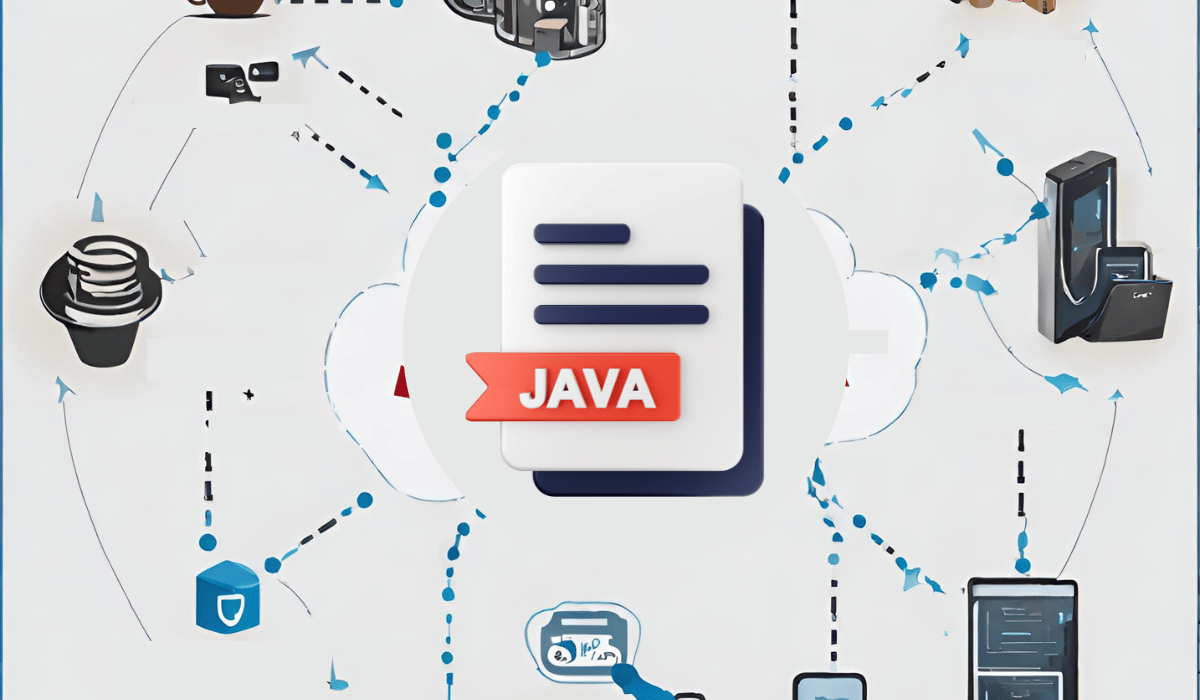
Before encrypting JSON data in Java, you must install the Java Development Kit (JDK) on your machine. The JDK includes the Java Runtime Environment (JRE) and additional tools and libraries required for Java development.
The JDK is a software development environment that provides the necessary tools and resources for developing Java applications. It includes the Java compiler, which compiles Java source code into bytecode that can be executed by the Java Virtual Machine (JVM). The JDK also includes various libraries and utilities that help developers build, debug, and deploy Java applications.
Installing the JDK is a straightforward process. You can download the JDK from the official Oracle website and follow the installation instructions provided. Once installed, you will have access to the tools and libraries required to encrypt JSON data in Java.
JSON Processing Library
You will need a JSON processing library to manipulate and process JSON data in Java. Several popular libraries are available, such as Gson, Jackson, and JSON-P. These libraries provide convenient methods for parsing, creating, and modifying JSON data.
Gson is a Java library developed by Google that provides simple and flexible APIs for converting Java objects to JSON and vice versa. It allows you to easily serialize and deserialize JSON data using Java classes and objects. Gson also supports custom serializers and deserializes, allowing you to handle complex JSON structures.
Jackson is another widely used JSON processing library for Java. It offers a high-performance JSON parser, generator, and powerful data binding capabilities. Jackson allows you to map JSON data to Java objects and vice versa, making it easy to work with JSON data in Java applications. It also supports advanced features such as JSON schema validation and streaming API.
JSON-P (JSON Processing) is a standard API for processing JSON data in Java. It is part of the Java EE platform and provides a set of interfaces and classes for working with JSON. JSON-P offers a simple and intuitive API for parsing, generating, and manipulating JSON data. It is lightweight and easy to use, making it a popular choice for developers who want a minimalistic JSON processing library.
When choosing a JSON processing library, consider factors such as performance, ease of use, and compatibility with your existing codebase. Each library has its strengths and features, so it’s important to evaluate them based on your specific requirements.
Step-by-Step Guide to Encrypt JSON Data in Java
Writing the Java Code
Start by creating a Java class that will handle the encryption of JSON data. This class will serve as the main entry point for the encryption process. Define the necessary variables and import the required libraries to ensure the encryption process runs smoothly.
When importing the libraries, choose the appropriate JSON processing library that best fits your needs. Several options are available, such as Jackson, Gson, and JSON.simple. Each library has its features and advantages, so choose the one that aligns with your project requirements.
Once you have imported the necessary libraries, you can use the chosen JSON processing library to parse the JSON data and store it in appropriate Java objects. This step is crucial as it allows you to manipulate the JSON data before encrypting it.
Implementing Encryption
Next, it’s time to implement the encryption process using a cryptographic algorithm. There are various encryption algorithms available, such as AES (Advanced Encryption Standard), RSA (Rivest-Shamir-Adleman), and DES (Data Encryption Standard). The choice of algorithm depends on your security requirements and the level of encryption you need.
For example, AES is a popular choice if you need a symmetric encryption algorithm. It offers strong security and is widely used in various applications. On the other hand, if you require asymmetric encryption, RSA is a commonly used algorithm. It provides a secure way to exchange encryption keys between parties.
Once you choose an appropriate algorithm, you must generate encryption keys. These keys are essential for the encryption and decryption process. Depending on the algorithm, you may need to generate a single key or a pair of keys (public and private keys).
With the encryption keys, you can now encrypt the JSON data. Use the chosen algorithm and the generated keys to encrypt the data. This process will transform the JSON data into a cipher text, making it unreadable to unauthorized parties.
Testing the Encryption
After implementing the encryption logic, it is crucial to verify its correctness by testing it with sample JSON data. Prepare a set of sample JSON data representing the kind of data you will encrypt in your application.
Encrypt the sample JSON data using the implemented encryption logic and ensure that the data is successfully encrypted. Once the data is encrypted, attempt to decrypt it using the appropriate keys and algorithm. This step is crucial to ensure the encryption process works as expected and the encrypted data can be decrypted correctly.
Thoroughly test the encryption process to ensure its reliability and accuracy. Test it with different JSON data types, including various data structures, nested objects, and arrays. This will help identify potential issues or edge cases that must be addressed.
Additionally, consider testing the performance of the encryption process. Measure the time it takes to encrypt and decrypt large JSON data sets to ensure the process is efficient and can handle the expected workload.
By following these steps and thoroughly testing the encryption process, you can ensure that your JSON data remains secure and protected from unauthorized access.
Common Errors and Troubleshooting
Debugging Your Code
If you encounter any issues during the encryption process, it is essential to debug your code effectively. Use debugging techniques, such as breakpoints and logging, to identify and resolve any errors or inconsistencies in your code—debugging aids in understanding the flow of execution and pinpointing problematic areas.
Common Encryption Issues
Encryption can sometimes be a complex process, and certain issues may arise. Some common encryption issues include key management, algorithm selection, and performance concerns. Ensure you use secure encryption algorithms and employ proper key management practices to mitigate potential risks.
Key Takeaways
- To encrypt JSON data in Java, you must use a suitable encryption algorithm, such as AES (Advanced Encryption Standard).
- Choose a secure key for encryption and ensure that it is properly protected.
- Convert the JSON data to a byte array or a string representation.
- Use the chosen encryption algorithm and the key to encrypt the JSON data.
- Serialize the encrypted data to a format suitable for storage or transmission, such as Base64 encoding.
- Apply appropriate padding and initialization vector (IV) to ensure secure encryption.
- Keep the encryption key secure and separate from the encrypted data.
- When decrypting the data, reverse the encryption process using the same algorithm, key, and IV.
FAQs
Why would I need to encrypt JSON data in Java?
Encrypting JSON data is essential when dealing with sensitive information that needs to be protected from unauthorized access. It ensures the confidentiality and integrity of the data during storage or transmission.
Can I use different encryption algorithms apart from AES?
While AES is a widely-used encryption algorithm, you can use other symmetric or asymmetric encryption algorithms available in Java’s cryptography libraries, such as RSA or Blowfish. The choice of algorithm depends on your specific requirements and security considerations.
How do I securely store the encryption key?
To store the encryption key securely, consider using a key management system or securely storing the key in a separate file with restricted access permissions. Avoid hardcoding the key in your code or storing it alongside the encrypted data.
Conclusion
By following these steps and embracing the fundamentals of encryption, you can effectively encrypt JSON data in Java. Encryption is a critical aspect of data protection, and understanding how to apply it to JSON data enables you to enhance the security and integrity of your applications. Take advantage of the tools and libraries available in the Java ecosystem to simplify the encryption process and ensure the confidentiality of your data.