You can encrypt data with ECDSA in Python by following these steps:
- Set up your Python environment by installing the necessary libraries, such as
ecdsa
.- Generate an ECDSA key pair consisting of a private key and a public key using the
ecdsa
library.- Encrypt data by signing it with the private key, which generates a digital signature.
- Decrypt data by verifying the digital signature using the public key.
In today’s digital age, data security is of paramount importance. Encryption is vital in protecting sensitive information from falling into the wrong hands.Together, we will delve into the world of encryption and explore the process of encrypting data with ECDSA (Elliptic Curve Digital Signature Algorithm) in Python. At the end of this , you get a solid understanding of how to implement this powerful encryption technique to ensure the security of your data.
Understanding Encryption and ECDSA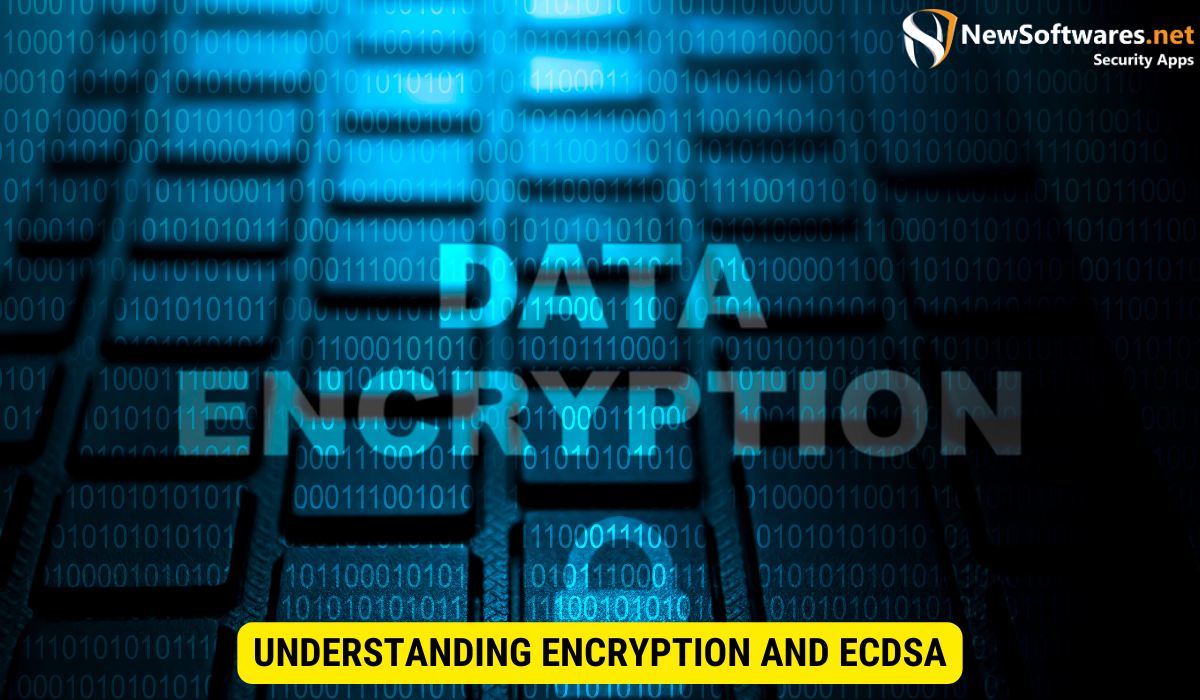
Encryption is the process of encrypting information so that only authorized parties can access it. It involves transforming plaintext (unencrypted data) into ciphertext (encrypted data) using an encoding algorithm and a secret key. The encrypted data can only be decrypted back into plaintext using the same key.
Encoding plays a vital role in ensuring the confidentiality and integrity of sensitive information. It is widely used in various applications, such as secure communication, data storage, and digital transactions. Without encryption, sensitive data would be vulnerable to unauthorized access and manipulation.
ECDSA, on the other hand, is a cryptographic algorithm used for digital signatures and encryption. It is based on elliptic curve mathematics, which provides a higher security level than traditional cryptographic algorithms.
The Basics of Encryption
Encryption works on the principle of using a secret key to transform data into an unreadable format. The process involves applying mathematical operations on the data using the key, making it incomprehensible to anyone without it.
There are two types of encryption algorithms, for instance, symmetric and asymmetric. Symmetric encoding uses the same key for both encryption and decryption. On the other hand, Asymmetric encryption utilizes a pair of keys – a free key for encryption and a private key for decryption.
In symmetric encryption, the top-secret key must be firmly shared between the sender and the recipient. This can be a challenge when the two parties have not established a secure key exchange channel. Asymmetric encryption addresses this challenge by using a pair of mathematically related keys.
As the name suggests, the public key can be freely shared with anyone. It is used by the sender to encrypt the data before transmission. On the other hand, the private key must be kept secret by the recipient. It is used for decrypting the data received using the corresponding public key.
What is ECDSA and How Does it Work?
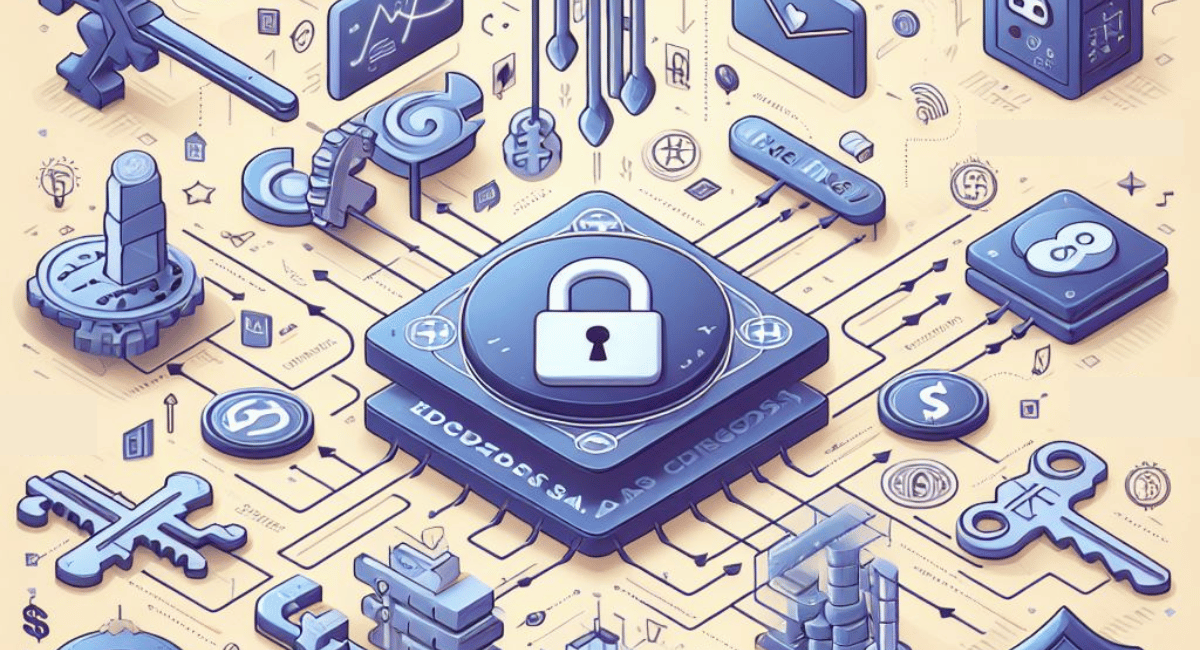
ECDSA is a public-key cryptography algorithm that provides secure digital signatures and encryption. It is based on the arithmetic of elliptic curves over finite fields. Unlike traditional RSA algorithms, ECDSA offers comparable security with shorter key lengths, making it more efficient.
The process of ECDSA involves generating a key pair: a private key and a corresponding free key. The private key is kept secret, while the free key is shared with others. The private key is used for signing data, while the public key is used for verification.
When signing data using ECDSA, the private key generates a digital signature. This signature serves as proof of the authenticity and integrity of the data. The signature can be verified using the consistent public key, ensuring that the data has not been tampered with and that it was indeed signed by the private key holder.
ECDSA also supports encryption by using the public key to encrypt data. The encrypted data can only be decrypted using the matching private key. This ensures that only the intended recipient possesses the private key and can access the encrypted information.
Overall, encryption and ECDSA are fundamental concepts in modern cryptography. They provide the necessary tools and techniques to secure sensitive information and enable secure communication and transactions in the digital world.
Setting Up Your Python Environment for ECDSA
Before we dive into the process of encrypting data with ECDSA in Python, we need to ensure that our Python environment is properly set up for ECDSA operations. This involves installing the necessary Python libraries and configuring them correctly.
Required Python Libraries for ECDSA
There are several Python libraries available that provide ECDSA functionality. One of the most popular ones is `ecdsa.` It is a pure Python implementation of the ECDSA algorithm and provides a simple and intuitive interface for working with ECDSA keys and encryption.
To install the `ecdsa` library, you can use the following command:
<code>pip install ecdsa</code>
Installing and Configuring ECDSA Libraries
Once you have installed the `ecdsa` library, you must configure it to work correctly with your Python environment. This involves importing the library into your Python script and setting up the necessary parameters for ECDSA operations.
Here is an example code snippet that demonstrates the basic setup for using the `ecdsa` library:
<code>import ecdsa# Generate a new ECDSA key pairprivate_key = ecdsa.SigningKey.generate(curve=ecdsa.SECP256k1)public_key = private_key.get_verifying_key()# Do further operations with the keys…</code>
The Process of Data Encryption Using ECDSA in Python
Now that we have our Python environment set up for ECDSA operations let’s explore the process of encrypting data using this powerful encryption technique.
Generating ECDSA Keys in Python
The first step in the encryption process is generating an ECDSA key pair. This key pair consists of a private key and a corresponding public key. The private key will be used for signing the data, while the public key will be used for verification.
In Python, we can use the `ecdsa` library to generate ECDSA keys with just a few lines of code:
<code>import ecdsa# Generate a new ECDSA key pairprivate_key = ecdsa.SigningKey.generate(curve=ecdsa.SECP256k1)public_key = private_key.get_verifying_key()</code>
Encrypting Data with ECDSA
Once we have our key pair, we can encrypt data using ECDSA. The process involves signing the data with the private key to generate a digital signature.
Here is an example code snippet that demonstrates the encryption process using ECDSA in Python:
<code>import ecdsa# Generate a new ECDSA key pairprivate_key = ecdsa.SigningKey.generate(curve=ecdsa.SECP256k1)public_key = private_key.get_verifying_key()# Encrypt data using ECDSAdata = “Hello, World!”signature = private_key.sign(data.encode())# Further operations with the signature…</code>
Decrypting Data with ECDSA
After encrypting the data using ECDSA, we can decrypt it by verifying the digital signature with the public key. If the signature is valid, the data has not been tampered with and can be safely decrypted.
Here is an example code snippet that demonstrates the decryption process using ECDSA in Python:
<code>import ecdsa# Generate a new ECDSA key pairprivate_key = ecdsa.SigningKey.generate(curve=ecdsa.SECP256k1)public_key = private_key.get_verifying_key()# Encrypt data using ECDSAdata = “Hello, World!”signature = private_key.sign(data.encode())# Decrypt data using ECDSAis_valid = public_key.verify(signature, data.encode())if is_valid: decrypted_data = “Data is valid: ” + data …else: decrypted_data = “Data is invalid.” …</code>
Common Errors and Troubleshooting in ECDSA Encryption
While working with ECDSA encryption, it is common to encounter errors and face challenges. In this section, we will discuss some common errors and provide best practices for troubleshooting.
Dealing with Common Encryption Errors
Some common errors that you might encounter while encrypting data with ECDSA include:
- Invalid key length error: This error occurs when the key length used for encryption does not match the expected length for the chosen curve. Use the correct key length for your ECDSA implementation to resolve this error.
- Signature verification failed: This error occurs when the signature generated during encryption does not match the one provided during decryption. Double-check the data, key, and encryption process to troubleshoot this error to ensure consistency.
- Key generation failure: This error occurs when the key generation process fails due to issues with the underlying cryptographic library or incorrect parameters. To resolve this error, ensure you use a reliable library and proper parameters for key generation.
Best Practices for Troubleshooting
When troubleshooting issues in ECDSA encryption, there are several best practices to keep in mind:
- Double-check all inputs and parameters: Ensure that all data, keys, and parameters used in the encryption process are correct and consistent. Even a small error can lead to encryption failures.
- Refer to the library documentation: If you encounter errors specific to the ECDSA library you are using, refer to the official documentation for troubleshooting guidance. Libraries often provide detailed information about common errors and their solutions.
- Seek help from the community: If you cannot resolve the issue alone, consider seeking help from the wider community. Online forums, discussion boards, and developer communities can provide valuable insights and solutions.
Enhancing Data Security with ECDSA Encryption
ECDSA encryption has become indispensable in ensuring data security in various applications. Its robustness, efficiency, and compatibility with modern cryptographic standards make it an excellent choice for protecting sensitive information.
The Role of ECDSA in Data Security
ECDSA plays a crucial role in data security by providing secure digital signatures and encryption. It offers strong cryptographic properties that make it virtually impossible for adversaries to tamper with encrypted data or impersonate authorized parties.
Advanced ECDSA Techniques for Enhanced Security
While the basic ECDSA encryption process is highly secure, there are advanced techniques that can further enhance the security of your encrypted data. These techniques include:
- Key Management: Implementing secure key management practices is essential to safeguarding ECDSA keys. This involves properly storing, rotating, and revoking keys when necessary.
- Hardware Security Modules (HSMs): Using HSMs can provide an additional layer of security by ensuring that the private keys are stored in tamper-resistant hardware, preventing unauthorized access.
- Multi-factor Authentication: Integrating multi-factor authentication methods, such as biometrics or smart cards, can add an extra layer of security to the encryption process.
By incorporating these advanced techniques into your data security strategy, you can significantly enhance the protection of your sensitive information.
Key Takeaways
- Encryption is vital for data security, and ECDSA (Elliptic Curve Digital Signature Algorithm) is a powerful encryption technique.
- ECDSA involves generating a key pair: a private key for signing and a public key for verification.
- Data is encrypted by signing it with the private key and decrypted by verifying the digital signature with the public key.
- Common errors in ECDSA encryption can be resolved by verifying inputs, consulting documentation, and seeking community help.
- Advanced techniques like key management, hardware security modules (HSMs), and multi-factor authentication can enhance data security.
FAQs
What is ECDSA encryption, and why is it important?
ECDSA (Elliptic Curve Digital Signature Algorithm) encryption is a cryptographic technique used to secure data by generating digital signatures and encrypting data. It’s important for protecting sensitive information and ensuring data integrity.
How does ECDSA encryption differ from other encryption methods?
ECDSA encryption is based on elliptic curve mathematics, providing strong security with shorter key lengths compared to traditional encryption methods like RSA.
What are the basic steps to encrypt data with ECDSA in Python?
The basic steps include setting up your Python environment, generating an ECDSA key pair, encrypting data by signing it with the private key, and decrypting data by verifying the digital signature with the public key.
What are some common errors in ECDSA encryption, and how can they be resolved?
Common errors include invalid key length, signature verification failures, and key generation problems. These errors can often be resolved by double-checking inputs, referring to library documentation, and seeking assistance from the community.
Are there advanced techniques to enhance ECDSA encryption security?
Yes, advanced techniques include secure key management, using Hardware Security Modules (HSMs), and implementing multi-factor authentication methods to further enhance data security.
Conclusion
In conclusion, we have explored the process of encrypting data with ECDSA in Python. We discussed the basics of encryption, the workings of ECDSA, and the steps involved in setting up a Python environment for ECDSA operations. We also covered the process of generating ECDSA keys, encrypting and decrypting data using ECDSA, and common errors and troubleshooting techniques in ECDSA encryption.
With the knowledge gained from this article, you are now equipped to implement ECDSA encryption in your Python projects and ensure the security of your data. Remember to follow best practices and stay updated with the latest advancements in data encryption to stay one step ahead of potential adversaries.