To encrypt data with Ruby:
-
Require OpenSSL:
require 'openssl'
in your Ruby script. -
Generate Key and IV: Use
key = OpenSSL::Cipher::AES.new(256, :CBC).encrypt.random_key
andiv = OpenSSL::Cipher::AES.new(256, :CBC).encrypt.random_iv
. -
Create Cipher: Initialize a cipher with
cipher = OpenSSL::Cipher::AES.new(256, :CBC).encrypt
. -
Encrypt Data: Use
cipher.update(data) + cipher.final
to encrypt your data.
In today’s digital age, the importance of data encryption cannot be overstated. With the increasing threat of hackers and cyber attacks, it has become crucial to protect sensitive information from falling into the wrong hands. Data encryption involves the process of converting plain text into an unreadable format, known as ciphertext, using various algorithms and keys. I will explore how to encrypt data using the powerful programming language Ruby.
Understanding Data Encryption
Before diving into the world of Ruby encryption, it is essential to grasp the significance of data encryption. The main objective of encryption is to provide confidentiality, integrity, and availability of data. By encrypting information, we can ensure that only authorized individuals can access and decipher it. Additionally, data encryption helps prevent tampering or modification during transmission or storage.
Data encryption plays a vital role in protecting sensitive information, such as personal details, financial transactions, and confidential business data. By encrypting data, organizations can comply with regulatory requirements, enhance customer trust, and safeguard their reputation. Encryption provides an extra layer of security, making it significantly more challenging for attackers to exploit vulnerabilities and gain unauthorized access to sensitive data.
When it comes to data encryption, there are several basic concepts that are important to understand. Encryption typically involves the use of algorithms and keys. Algorithms, such as Advanced Encryption Standard (AES) or RSA, determine the encryption and decryption processes. These algorithms use complex mathematical calculations to transform plaintext data into ciphertext and vice versa. The choice of algorithm depends on factors such as the level of security required and the computational resources available.
Keys, on the other hand, are unique values that govern the encryption and decryption processes. There are two types of keys: symmetric and asymmetric. Symmetric encryption uses the same key for both encryption and decryption, while asymmetric encryption uses a pair of keys – a public key for encryption and a private key for decryption. The use of asymmetric encryption allows for secure communication between parties who have never met or shared a key before.
In addition to algorithms and keys, data encryption also involves other important concepts such as key management, key exchange, and digital signatures. Key management refers to the processes and procedures for generating, storing, distributing, and revoking encryption keys. Key exchange is the process of securely sharing encryption keys between parties. Digital signatures, on the other hand, are used to verify the authenticity and integrity of digital documents or messages.
Overall, understanding data encryption is crucial in today’s digital age where the protection of sensitive information is of utmost importance. By implementing robust encryption practices, organizations can ensure the confidentiality, integrity, and availability of their data, thereby mitigating the risks associated with unauthorized access and data breaches.
Introduction to Ruby for Encryption
Ruby is a versatile programming language known for its simplicity and elegance. It offers several features that make it an excellent choice for data encryption purposes. Let’s explore why Ruby is a popular choice for encryption-related tasks.
When it comes to encryption, Ruby shines with its intuitive syntax and expressive nature. The language’s flexibility allows for efficient implementation of encryption algorithms, making it a favorite among developers. Ruby’s object-oriented design further enhances its appeal, as it facilitates code organization and modularity, making it easier to manage encryption-related functionalities.
But what sets Ruby apart from other programming languages for encryption? One of the key features that make Ruby a top choice is its high-level abstractions for cryptographic operations. These abstractions allow developers to focus on the core encryption logic rather than getting bogged down in low-level implementation details. This not only saves time but also makes the code more readable and maintainable.
Another advantage of using Ruby for encryption is its built-in support for multi-threading and concurrency. Encryption tasks often require high performance, and Ruby’s concurrency capabilities make it well-suited for such scenarios. With Ruby, developers can leverage the power of parallel processing to speed up encryption operations, ensuring optimal performance even with large volumes of data.
Furthermore, Ruby’s open-source nature is a significant advantage for encryption-related tasks. The vibrant Ruby community has developed numerous libraries and frameworks specifically designed for encryption. These community-driven resources provide developers with a wealth of options and tools to enhance their encryption capabilities. Whether it’s implementing advanced encryption algorithms or integrating with existing encryption protocols, Ruby’s open-source ecosystem has got you covered.
In conclusion, Ruby’s simplicity, elegance, and powerful features make it an excellent choice for encryption-related tasks. Its intuitive syntax, high-level abstractions, support for multi-threading, and extensive library ecosystem all contribute to its popularity among developers. So, if you’re looking to dive into the world of encryption, Ruby is definitely worth considering.
Why Use Ruby for Encryption?
Ruby’s intuitive syntax and expressive nature make it an appealing choice for developers interested in encryption. The language’s flexibility allows for efficient implementation of encryption algorithms and provides an extensive library ecosystem for cryptography-related tasks. Ruby’s object-oriented design also facilitates code organization and modularity, making it easier to manage encryption-related functionalities.
Key Features of Ruby for Encryption
Ruby provides a range of features that significantly simplify the process of data encryption. Firstly, Ruby offers high-level abstractions for cryptographic operations, allowing developers to focus on the core encryption logic rather than low-level implementation details. Additionally, Ruby’s built-in support for multi-threading and concurrency makes it well-suited for performance-critical encryption tasks. Finally, Ruby’s open-source nature means that developers can benefit from community-driven libraries and frameworks specifically designed for encryption.
Setting up Your Ruby Environment
Before getting started with encryption using Ruby, it is essential to set up your development environment to ensure a smooth experience.
Setting up your Ruby environment involves installing Ruby and necessary tools and libraries that can enhance your encryption experience.
Installing Ruby
To use Ruby for encryption, you need to have Ruby installed on your machine. Ruby is a dynamic, open-source programming language known for its simplicity and flexibility.
Installing Ruby is a straightforward process. You can download the appropriate installer for your operating system from the official Ruby website and follow the installation instructions. Alternatively, if you prefer using a package manager, most operating systems provide a way to install Ruby through their respective package managers.
Once installed, you can verify your Ruby installation by typing ruby -v in your command prompt or terminal. This command will display the version of Ruby installed on your machine.
Necessary Tools and Libraries
In addition to Ruby itself, there are a few additional tools and libraries that can enhance your encryption experience.
One such critical library is ‘openssl’, which provides a comprehensive set of cryptographic functions and algorithms. OpenSSL is widely used in the industry for secure communication, data encryption, and digital certificates.
To install ‘openssl’, you can make use of Ruby’s package manager, RubyGems. RubyGems is a package manager that allows you to easily install and manage Ruby libraries and dependencies.
To install ‘openssl’ using RubyGems, open your command prompt or terminal and run the following command:
gem install openssl
This command will download and install the ‘openssl’ library, making it available for use in your Ruby programs.
Once installed, you can require the ‘openssl’ library in your Ruby code to access its cryptographic functions and algorithms.
By setting up your Ruby environment with the necessary tools and libraries, you are now ready to explore the world of encryption using Ruby. Whether you are encrypting sensitive data, securing communication channels, or implementing secure authentication mechanisms, Ruby provides a robust and flexible platform for all your encryption needs.
Writing Your First Encryption Program with Ruby
Now that we have our Ruby environment set up let’s dive into writing our first encryption program. Starting with basic knowledge of Ruby syntax, we will gradually build up a fully functional encryption program.
Understanding Ruby Syntax
Before writing our first encryption program, let’s explore some essential Ruby syntax. Ruby is known for its readability and simplicity, which makes it an excellent choice for beginners.
Step-by-step Guide to Writing an Encryption Program
Now that we have a basic understanding of Ruby syntax, let’s dive into writing our encryption program. In this step-by-step guide, we will cover the necessary steps to create a simple encryption program using Ruby. We will start by importing the necessary libraries and defining our encryption algorithm and key. Then, we will write functions for encryption and decryption and, finally, test our program using sample input.
Exploring Different Encryption Algorithms
Ruby provides support for various encryption algorithms, each with its unique characteristics and purposes. Let’s explore two common types of encryption: symmetric encryption and asymmetric encryption.
Symmetric Encryption in Ruby
Symmetric encryption, also known as secret-key encryption, involves using the same key for both encryption and decryption. This type of encryption is well-suited for scenarios where speed and efficiency are crucial. Ruby provides several symmetric encryption algorithms, such as AES and DES, which offer varying levels of security and performance.
Asymmetric Encryption in Ruby
Unlike symmetric encryption, asymmetric encryption, also known as public-key encryption, uses different keys for encryption and decryption. Asymmetric encryption provides enhanced security and is widely used in scenarios where secure key exchange and digital signatures are required. Ruby supports popular asymmetric encryption algorithms, such as RSA and DSA, which offer robust security features.
Key Takeaways
- Data encryption is crucial for protecting sensitive information and ensuring data confidentiality.
- Ruby’s simplicity, high-level abstractions, and library ecosystem make it an excellent choice for encryption tasks.
- Setting up your Ruby environment for encryption involves installing Ruby and the ‘openssl’ library using RubyGems.
- Ruby supports both symmetric and asymmetric encryption algorithms, providing flexibility for different security needs.
- By following the step-by-step guide and exploring encryption algorithms, you can enhance data security using Ruby.
FAQs
1. What is data encryption, and why is it important?
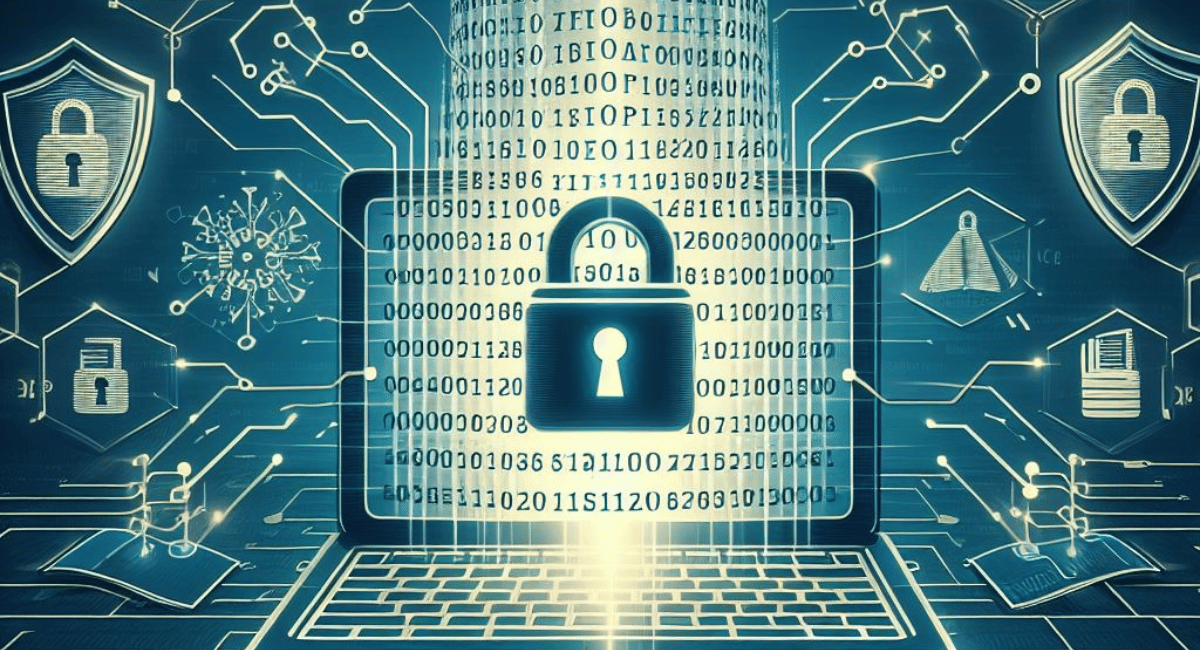
Data encryption is the process of converting plain text into unreadable ciphertext to protect sensitive information. It’s essential for ensuring data confidentiality and preventing unauthorized access.
2. Why choose Ruby for data encryption?
Ruby’s simplicity, high-level abstractions, and extensive library ecosystem make it a popular choice for encryption tasks, offering readability and efficiency.
3. What are the key features of Ruby for encryption?
Ruby provides high-level abstractions for cryptographic operations, support for multi-threading, and an open-source ecosystem of libraries and frameworks for encryption.
4. How do I set up my Ruby environment for encryption?
Install Ruby and essential libraries like ‘openssl’ using RubyGems to set up your development environment for encryption tasks.
5. What are the different encryption algorithms available in Ruby?
Ruby supports both symmetric encryption (e.g., AES, DES) and asymmetric encryption (e.g., RSA, DSA), offering flexibility for various security requirements.
Conclusion
Data encryption is a critical component of cybersecurity, and Ruby’s features and libraries make it a valuable tool for implementing encryption solutions. By understanding the importance of data encryption, setting up your Ruby environment, and exploring encryption algorithms, you can effectively protect sensitive information and enhance the security of your applications. Stay tuned for more articles on data encryption and Ruby programming.