What is encryption in Python?
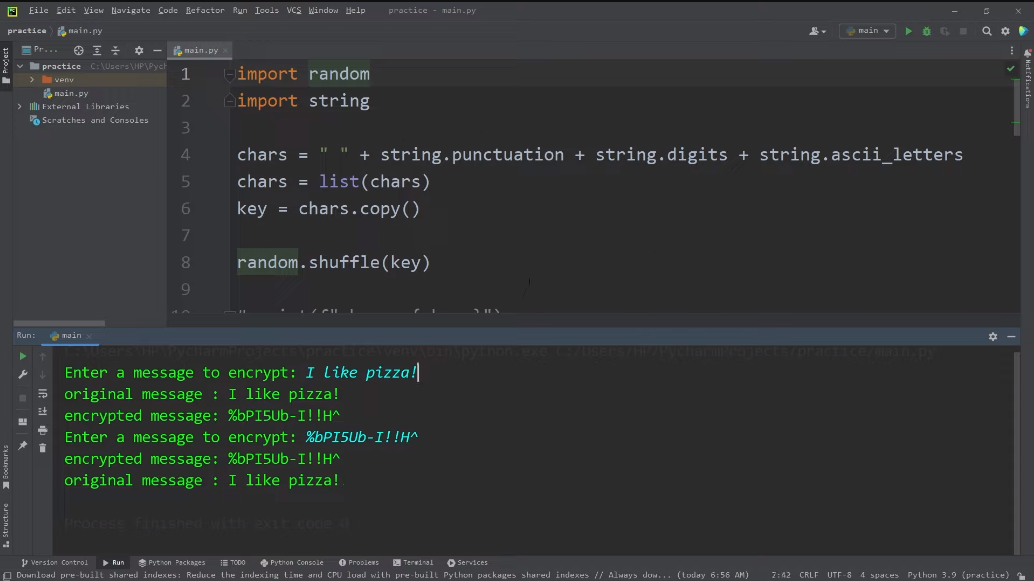
Encryption in Python refers to the process of converting plain text or data into a coded form to protect its confidentiality and integrity. There are various encryption algorithms available in Python, such as AES, DES, RSA, and Blowfish, among others. These algorithms use different techniques to scramble the data, making it unreadable without the proper decryption key. Encryption is commonly used in secure communication, data storage, and password protection.
Benefits of Using Encryption In Python
There are several benefits of using encryption in Python, including:
1. Confidentiality: Encryption ensures that only authorized individuals can access confidential information. This is particularly important for sensitive data such as financial information, personal data, and intellectual property.
2. Data integrity: Encryption ensures that data remains unchanged during transmission or storage. This is important to prevent unauthorized modification of data, which can compromise its validity and accuracy.
Popular Symmetric Encryption Algorithms In Python
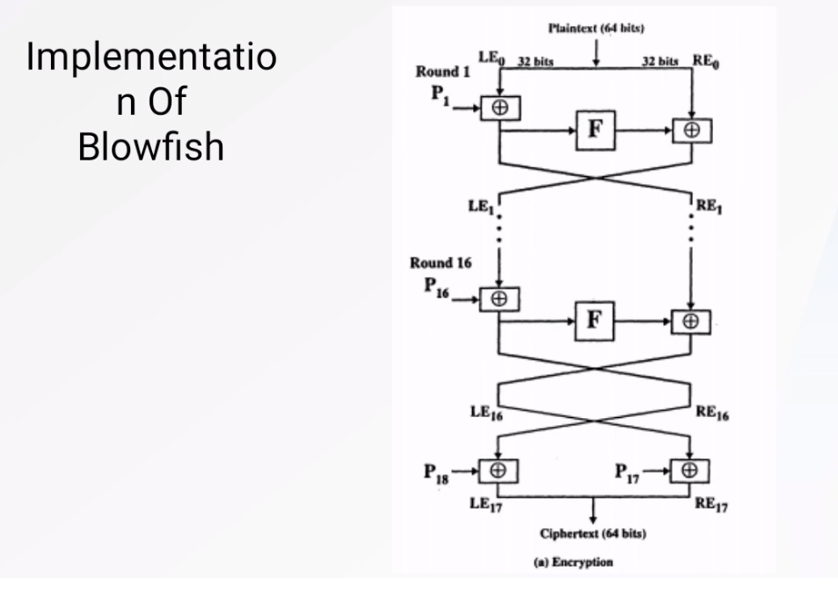
There are several popular symmetric encryption algorithms available in Python, including:
1. Advanced Encryption Standard (AES): This is a widely-used encryption algorithm that is known for its high level of security and efficiency. It uses a symmetric key to encrypt and decrypt data.
2. Blowfish: This is another popular symmetric encryption algorithm that is known for its speed and flexibility. It can be used with key sizes ranging from 32 bits to 448 bits.
Popular Asymmetric Encryption Algorithms In Python
There are several popular asymmetric encryption algorithms available in Python, including:
1. RSA: This is one of the most widely-used asymmetric encryption algorithms. It uses a public key and a private key to encrypt and decrypt data. RSA is known for its security and is commonly used for securing communication over the internet.
2. Elliptic Curve Cryptography (ECC): This is a newer asymmetric encryption algorithm that is becoming increasingly popular due to its efficiency and security. ECC uses elliptic curves to generate public
Hashing and Encryption
Hashing and encryption are two important concepts in cryptography. Hashing is a process of converting data of any size into a fixed-size output, which is called a hash. The hash is a unique representation of the original data, and any modification to the data will result in a different hash. Hashing is commonly used for data integrity checks, password storage, and digital signatures. Encryption, on the other hand, is a process of transforming data into a form that is unreadable without a key.
Difference Between Hashing And Encryption
Hashing and encryption are both important concepts in cryptography, but they serve different purposes. Hashing is a one-way process of converting data into a fixed-size output, which is a unique representation of the original data. The hash cannot be reversed to obtain the original data, and any modification to the data will result in a different hash. Hashing is commonly used for data integrity checks, such as verifying that a file has not been tampered with or corrupted.
Popular Data-At-Rest Encryption Techniques In Python
There are several popular data-at-rest encryption techniques in Python, including:
1. Advanced Encryption Standard (AES): This is a symmetric-key encryption algorithm that uses a block cypher to encrypt and decrypt data. AES is widely used and considered to be secure.
2. Blowfish: This is another symmetric-key encryption algorithm that uses a block cypher. It is popular for its speed and efficiency, but it is not as widely used as AES.
Popular Password Encryption Techniques In Python
There are several popular password encryption techniques in Python, including:
1. PBKDF2: This is a key derivation function that is used to generate a cryptographic key from a password. It is widely used and considered to be secure.
2. bcrypt: This is a password hashing function that is designed to be slow and computationally expensive, making it difficult for attackers to crack passwords.
3. script: This is another password hashing function that is designed to be memory-intensive, making it difficult for
Conclusion
In Python, there are several popular password encryption techniques that can be used to secure passwords. PBKDF2, bcrypt, and scrypt are all good options for password encryption, and each has its own strengths and weaknesses. It’s important to choose the right encryption technique based on the specific needs of your application and the level of security you require. Remember, the best way to protect passwords is to use strong encryption techniques and to encourage users to choose strong, unique passwords.
FAQs
What are some popular encryption algorithms in Python?
Some popular encryption algorithms in Python include Advanced Encryption Standard (AES), Data Encryption Standard (DES), Blowfish, and Twofish. These algorithms can be used to encrypt data for secure transmission or storage. It’s important to choose the right algorithm based on the specific needs of your application and the level of security you require.
How do I implement encryption in Python?
Implementing encryption in Python depends on the specific encryption algorithm you choose. Generally, you will need to import the appropriate encryption library, generate a key, and use that key to encrypt and decrypt your data. Here is a general outline of the steps you would take:
1. Choose an encryption algorithm that fits your needs.
2. Install the library for that algorithm (if necessary).
3. Generate a key using a secure method.
4. Use the key to encrypt your data.
How do I combine hashing and encryption in Python?
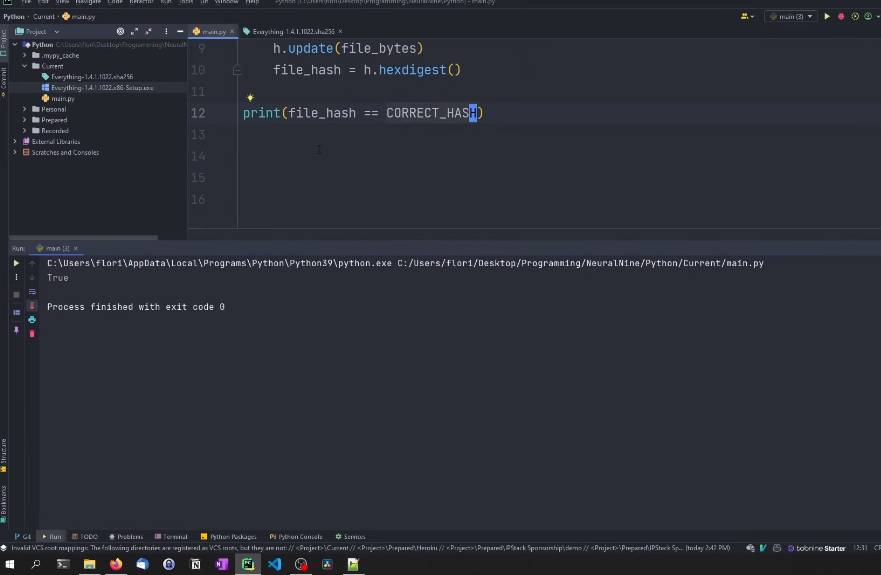
Combining hashing and encryption can provide an additional layer of security to your data. Here is a general outline of the steps you would take to combine hashing and encryption in Python:
1. Choose a hashing algorithm that fits your needs and import the appropriate library (such as Hashlib).
2. Generate a hash of your data using the chosen algorithm.
3. Choose an encryption algorithm and import the appropriate library (such as cryptography).
4. Generate a key using a secure method.